The term “named route” is used to give a route a specific name. Using the “as” array key, the name can be assigned.
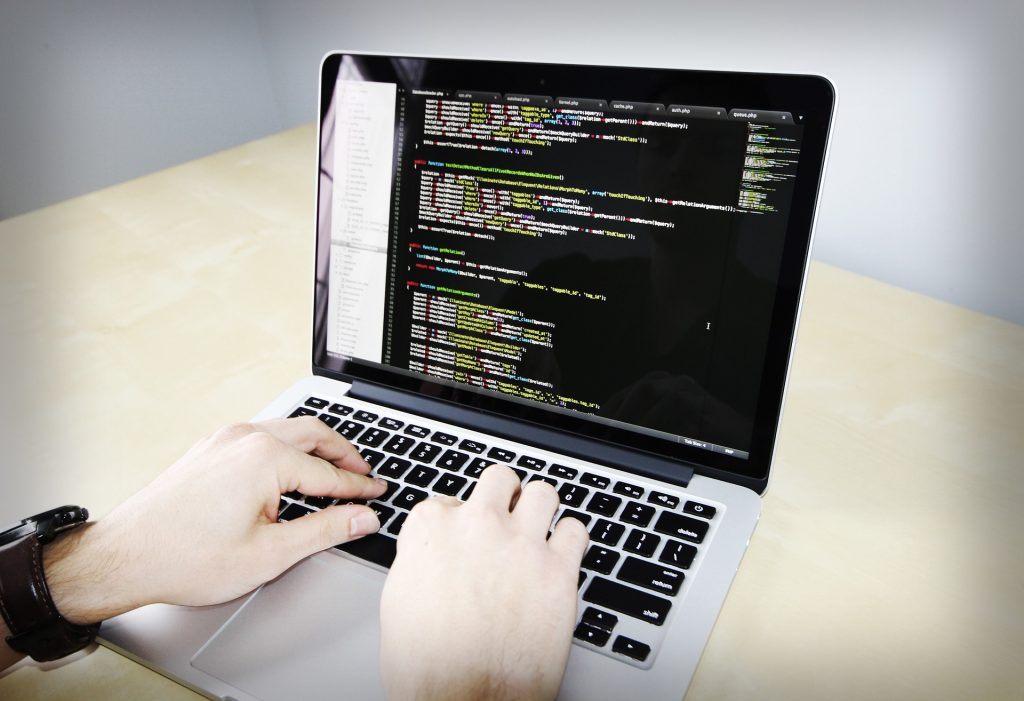
Route::get('user/profile', ['as' => 'profile', function () {
//
}]);
Note: The user/profile route has been given the name profile.
Example
The following example demonstrates redirecting to named routes.
Step 1: Put a new view file, test.php here
Views/resources/test.php.
<html>
<body>
<h1>Example of Redirecting to Named Routes</h1>
</body>
</html>
Step 2: We have configured the route for the test.php file in routes.php. It is now called testing. In order to reroute the request to the designated route testing, we have additionally established an additional route redirect.
app/Http/routes.php
Route::get('/test', ['as'=>'testing',function() {
return view('test2');
}]);
Route::get('redirect',function() {
return redirect()->route('testing');
});
Step 3: To test the named route example, go to the following URL.
http://localhost:8000/redirect
Step 4: You will be redirected to http://localhost:8000/test after the aforementioned URL has been executed. This is because we are redirecting to the designated route testing.
Step 5: The following output will appear once the URL has been successfully executed.
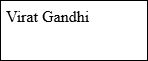
Taking you to Controller Actions
We have the ability to redirect to controller actions in addition to defined routes. The action method only has to be sent the controller and action name, as the example below illustrates. A parameter can be sent as the action method’s second argument if you wish to do so.
return redirect()->action(‘NameOfController@methodName’,[parameters]);
Example
Step 1 – To create a controller named RedirectController, run the following command.
php artisan make:controller RedirectController --plain
Step 2 – The following output will be obtained upon successful completion-

Step 3: Copy and save the code below.
Controllers/RedirectController.php in app/Http
DirectController.php is located in app/Http/Controllers.
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use App\Http\Requests;
use App\Http\Controllers\Controller;
class RedirectController extends Controller {
public function index() {
echo "Redirecting to controller's action.";
}
}
Step 4: Include the lines below in app/Http/routes.php.
Route::get('rr','RedirectController@index');
Route::get('/redirectcontroller',function() {
return redirect()->action('RedirectController@index');
});
Step 5: To test the example, go to the URL below.
http://localhost:8000/redirectcontroller
Step 6: The output will look like the picture below.
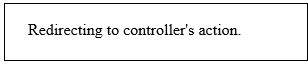