Middleware serves as an intermediary between the request and the response, functioning as a filtering tool. This section will guide you through the middleware process in Laravel. Laravel provides middleware that checks if the user is authenticated. If the user is logged in, they are redirected to the homepage; otherwise, they are directed to the login page.
Middleware is created by executing the following command:
php artisan make:middleware <middleware-name>
After replacing <middleware-name> with your chosen name, the middleware will be saved in the app/Http/Middleware directory.
Example:
The following example illustrates the middleware mechanism.
Step 1: Create AgeMiddleware by running this command:
php artisan make:middleware AgeMiddleware
Step 2: The following output indicates successful command execution:
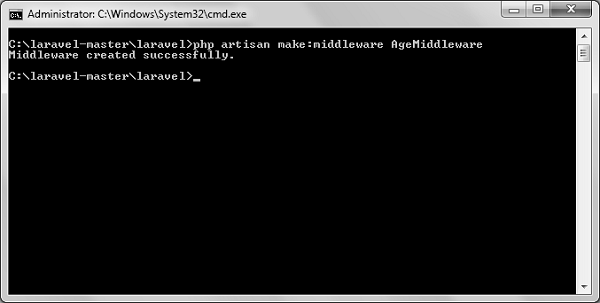
Step 3: The AgeMiddleware file, created in app/Http/Middleware, will already contain this code:
<?php
namespace App\Http\Middleware;
use Closure;
class AgeMiddleware {
public function handle($request, Closure $next) {
return $next($request);
}
}
The Middleware Registration Process
The use of middleware in Laravel necessitates prior registration. Two types of middleware are available within the framework.
- Globally Registered Middleware
- Route-Based Middleware
While the Route Middleware will be linked to a particular route, the Global Middleware will execute in response to each HTTP request made by the application. You can register the middleware under app/Http/Kernel.php. The properties $middleware
and $routeMiddleware
are present in this file. Global middleware is registered using the $middleware
property, while route-specific middleware is registered using the $routeMiddleware
property.
Global middleware registration is accomplished by appending the middleware class to the $middleware
property.
protected $middleware = [
\Illuminate\Foundation\Http\Middleware\CheckForMaintenanceMode::class,
\App\Http\Middleware\EncryptCookies::class,
\Illuminate\Cookie\Middleware\AddQueuedCookiesToResponse::class,
\Illuminate\Session\Middleware\StartSession::class,
\Illuminate\View\Middleware\ShareErrorsFromSession::class,
\App\Http\Middleware\VerifyCsrfToken::class,
];
Route-specific middleware registration involves adding a key-value pair to the $routeMiddleware
property.
protected $routeMiddleware = [
'auth' => \App\Http\Middleware\Authenticate::class,
'auth.basic' => \Illuminate\Auth\Middleware\AuthenticateWithBasicAuth::class,
'guest' => \App\Http\Middleware\RedirectIfAuthenticated::class,
];
Example
In the last example, we developed AgeMiddleware. It can now be registered in the middleware property that is particular to the route. Below is the code for that registration.
Below is the code found in app/Http/Kernel.php
<?php
namespace App\Http;
use Illuminate\Foundation\Http\Kernel as HttpKernel;
class Kernel extends HttpKernel {
protected $middleware = [
\Illuminate\Foundation\Http\Middleware\CheckForMaintenanceMode::class,
\App\Http\Middleware\EncryptCookies::class,
\Illuminate\Cookie\Middleware\AddQueuedCookiesToResponse::class,
\Illuminate\Session\Middleware\StartSession::class,
\Illuminate\View\Middleware\ShareErrorsFromSession::class,
\App\Http\Middleware\VerifyCsrfToken::class,
];
protected $routeMiddleware = [
'auth' => \App\Http\Middleware\Authenticate::class,
'auth.basic' => \Illuminate\Auth\Middleware\AuthenticateWithBasicAuth::class,
'guest' => \App\Http\Middleware\RedirectIfAuthenticated::class,
'Age' => \App\Http\Middleware\AgeMiddleware::class,
];
}
Middleware Parameterization
We can also pass parameters through the middleware. For instance, you can use middleware to pass parameters if your application has many roles, such as user, admin, super admin, etc., and you wish to authenticate the action according to role. We may pass our own argument after the $next
argument in the middleware we develop, which has the following function.
public function handle($request, Closure $next) {
return $next($request);
}
Example
Step 1: RoleMiddleware
is created by executing the following command:
php artisan make:middleware RoleMiddleware
Step 2: Upon successful completion, the output will be:
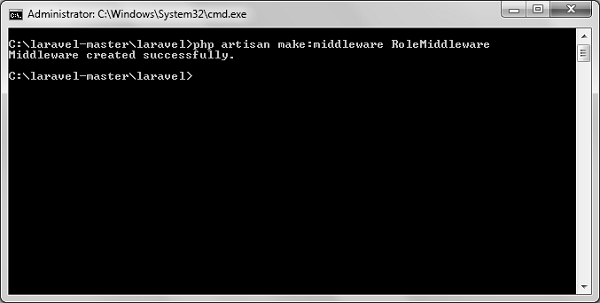
Step 3: In the RoleMiddleware.php file (app/Http/Middleware/RoleMiddleware.php), add the following code to the handle
method:
<?php
namespace App\Http\Middleware;
use Closure;
class RoleMiddleware {
public function handle($request, Closure $next, $role) {
echo "Role: ".$role;
return $next($request);
}
}
Step 4: Register RoleMiddleware within the app/Http/Kernel.php file by including the indicated (gray-highlighted) line of code.

Step 5: Use this command to generate TestController
php artisan make:controller TestController --plain
Step 6: Successful execution yields the following output:

Step 7: Paste the following code into app/Http/TestController.php
app/Http/TestController.php
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use App\Http\Requests;
use App\Http\Controllers\Controller;
class TestController extends Controller {
public function index() {
echo "<br>Test Controller.";
}
}
Step 8: Insert the following code into app/Http/routes.php
app/Http/routes.php
Route::get('role',[
'middleware' => 'Role:editor',
'uses' => 'TestController@index',
]);
Step 9: he following URL may be used to test the middleware’s parameter functionality:
http://localhost:8000/role
Step 10: The output will look like the picture below.
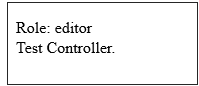
Middleware with Termination Capability
Terminable middleware executes code after a response is sent to the browser. This is done by adding a terminate method to your middleware class, which should then be registered as global middleware. The terminate method receives the request and response objects ($request
, $response
) as arguments. Here’s an example of how to create this method:
Example
Step 1: TerminateMiddleware is created by executing the following command:
php artisan make:middleware TerminateMiddleware
Step 2: The command will produce the following output:
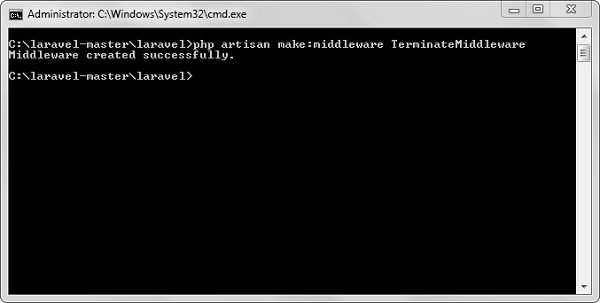
Step 3: The app/Http/Middleware/TerminateMiddleware.php file should contain the following code
<?php
namespace App\Http\Middleware;
use Closure;
class TerminateMiddleware {
public function handle($request, Closure $next) {
echo "Executing statements of handle method of TerminateMiddleware.";
return $next($request);
}
public function terminate($request, $response) {
echo "<br>Executing statements of terminate method of TerminateMiddleware.";
}
}
Step 4: To register TerminateMiddleware, include the highlighted line in the app/Http/Kernel.php file.

Step 5: Use this command to generate ABCController
php artisan make:controller ABCController --plain
Step 6: Successful execution of the URL request yields the following output:

Step 7: The app/Http/TestController.php file should contain the following code:
app/Http/TestController.php
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use App\Http\Requests;
use App\Http\Controllers\Controller;
class TestController extends Controller {
public function index() {
echo "<br>Test Controller.";
}
}
Step 8: The app/Http/routes.php file should include the following line of code:
app/Http/routes.php
Route::get('role',[
'middleware' => 'Role:editor',
'uses' => 'TestController@index',
]);
Step 9: The following URL can be used to test the middleware's parameter functionality:
http://localhost:8000/role
Step 10: You should see the following output:
