Cookies are crucial to a user’s experience using a web application. Working with cookies in Laravel-based web applications is covered in this chapter.
The Laravel global cookie helper can be used to create cookies. It is a Symfony\Component\HttpFoundation\Cookie instance. The withCookie() method can be used to attach the cookie to the response. To invoke the withCookie() method, create a response instance of the Illuminate\Http\Response class. The client cannot alter or access the encrypted and signed cookies created by Laravel.
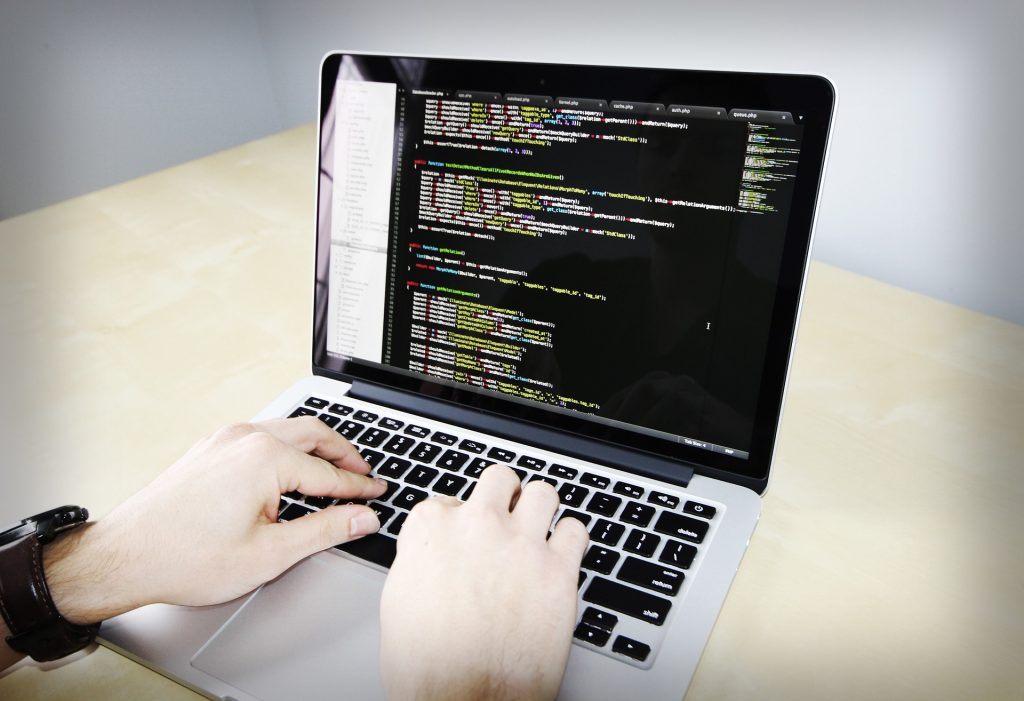
This code sample includes an explanation.
//Create a response instance
$response = new Illuminate\Http\Response('Hello World');
//Call the withCookie() method with the response method
$response->withCookie(cookie('name', 'value', $minutes));
//return the response
return $response;
The Cookie() function requires three arguments. The cookie name is the first argument; its value is the second; and its duration—the amount of time after which it will be automatically deleted—is the third.
As demonstrated in the code below, the forever method can be used to set a cookie for all time.
$response->withCookie(cookie()->forever('name', 'value'));
Retrieving Cookie Data
The cookie() method allows us to get the cookie once it has been set. The cookie’s name is the only argument that this cookie() function will accept. The Illuminate\Http\Request object can be used to invoke the cookie method.
This is an example of code.
//’name’ is the name of the cookie to retrieve the value of
$value = $request->cookie('name');
Example
Take a look at this example to learn more about cookies.
Step 1 − To construct a controller in which we will manipulate the cookie, run the command below.
php artisan make:controller CookieController --plain
Step 2 − You will obtain the following output following a successful execution.

Step 3 − Copy the code below.
app/Http/Controllers/CookieController.php file.
app/Http/Controllers/CookieController.php
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use Illuminate\Http\Response;
use App\Http\Requests;
use App\Http\Controllers\Controller;
class CookieController extends Controller {
public function setCookie(Request $request) {
$minutes = 1;
$response = new Response('Hello World');
$response->withCookie(cookie('name', 'virat', $minutes));
return $response;
}
public function getCookie(Request $request) {
$value = $request->cookie('name');
echo $value;
}
}
Step 4− In the app/Http/routes.php file, add the line that follows.
app/Http/routes.php
Route::get('/cookie/set','CookieController@setCookie');
Route::get('/cookie/get','CookieController@getCookie');
Step 5 − To set the cookie, go to the following URL.
http://localhost:8000/cookie/set
Step 6 − The output will look like this. Although the screenshot shows a dialog from Firefox, you can verify cookies from the cookie option in your browser.

Step 7 − To obtain the cookie from the aforementioned URL, go to the following URL.
http://localhost:8000/cookie/get
Step 8 − The output will look like the picture below.

Laravel Responses
Laravel has a variety of methods for returning responses. Either the route or the controller can send the response. Simple strings, as seen in the sample code below, are the most basic answer that may be given. This string will be transformed into the proper HTTP response automatically.
Example
Step 1 − add the code below to the app/Http/routes.php file.
app/Http/routes.php
Route::get('/basic_response', function () {
return 'Hello World';
});
Step 2 − To test the basic response, go to the following URL.
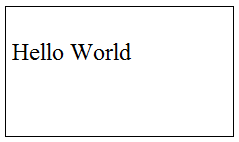
Attaching Response Headers
The header() method can be used to connect the response to headers. As seen in the sample code below, we may also attach the set of headers.
return response($content,$status)
->header('Content-Type', $type)
->header('X-Header-One', 'Header Value')
->header('X-Header-Two', 'Header Value');
Example
Take a look at this sample to learn more about response.
Step 1 − Include the following code in the file app/Http/route.php.
app/Http/route.php
Route::get('/header',function() {
return response("Hello", 200)->header('Content-Type', 'text/html');
});
Step 2 − To test the basic response, go to the following URL.
http://localhost:8000/header
Step 3 − The outcome will be as seen in the image below.
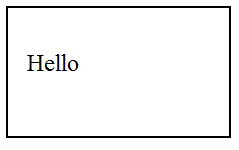
Adding Cookies
Cookies are attached using the withcookie() helper function. The withcookie() function can be called with a response object to attach the cookie created using this method. All of the cookies created by Laravel are by default signed and encrypted to prevent client modification or reading.
Step 1 − Include the following code in the file app/Http/routes.php.
app/Http/routes.php
Route::get('/cookie',function() {
return response("Hello", 200)->header('Content-Type', 'text/html')
->withcookie('name','Virat Gandhi');
});
Step 2 − To test the basic response, go to the following URL.
http://localhost:8000/cookie
Step 3 − The outcome will be as seen in the image below.
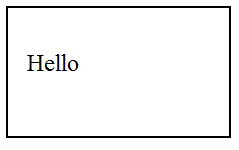
JSON Response Handling
The json method can be used to send a JSON response. The Content-Type header will be automatically set to application/json using this method. The array will be automatically converted into the proper JSON answer by the json method.
Example
To learn more about JSON Response, look at the example that follows.
Step 1 − Include the following code in the file app/Http/routes.php.
app/Http/routes.php
Route::get('json',function() {
return response()->json(['name' => 'Virat Gandhi', 'state' => 'Gujarat']);
});
Step 2 − To test the json response, go to the following URL.
http://localhost:8000/json
Step 3 − The outcome will be as seen in the image below.
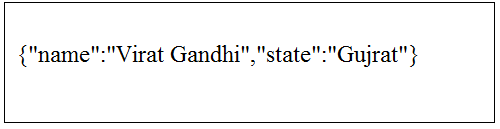