jQuery’s noConflict()
method is your solution. It gracefully releases jQuery’s hold on the $
symbol, allowing you to use a different alias (like jQuery) for jQuery’s functions, preventing the conflict with other libraries. This ensures both libraries can work together harmoniously on the same page without stepping on each other’s toes. This is crucial for maintaining website stability and preventing unexpected errors. You then use jQuery instead of $
to call jQuery functions.

jQuery and Other JavaScript Frameworks
The $ symbol is used by jQuery as a shortcut, as you are already aware.
Numerous additional well-known JavaScript frameworks exist, including Knockout, Ember, Backbone, Angular, and others.
What if other JavaScript frameworks also use the $ sign as a shortcut?
One framework may stop functioning if two other frameworks use the same shortcut.
This has already been considered and the noConflict() method has been developed by the jQuery team.
Understanding jQuery’s noConflict() Method
To allow other scripts to utilize the $ shortcut identifier, the noConflict() method releases its hold.
Of course, you can still utilize jQuery by just entering the entire name rather than the shortcut:
Example
$.noConflict();
jQuery(document).ready(function(){
jQuery("button").click(function(){
jQuery("p").text("jQuery is still working!");
});
});
It’s also quite easy to make your own shortcut. A reference to jQuery is returned by the noConflict() method, which you can store in a variable for subsequent usage. Here’s an illustration:
Example
var jq = $.noConflict();
jq(document).ready(function(){
jq("button").click(function(){
jq("p").text("jQuery is still working!");
});
});
You can pass the $
sign in as a parameter to the ready method if you have a block of jQuery code that uses the $
shortcut and you don’t want to modify it all. Inside this method, you can use $
to access jQuery; outside of it, you must use “jQuery”:
Example
$.noConflict();
jQuery(document).ready(function($){
$("button").click(function(){
$("p").text("jQuery is still working!");
});
});
Using jQuery Filters
jQuery’s filter methods go far beyond basic selectors. They’re not just about finding elements; they’re about intelligently refining your selection based on complex criteria. Imagine you have a list of users, and you only want to highlight those who are active and subscribed. Simple selectors won’t cut it. jQuery filters, however, allow you to chain conditions, creating highly specific selections. This precision is crucial for efficient DOM manipulation, ensuring you only target the elements you need, leading to cleaner, more performant code and avoiding accidental modifications. This targeted approach is key to building dynamic and responsive user interfaces.
Debugging mode is set to false by default, however it can be changed to true. This allows the user to use stack traces to track all problems.
Search for entries in a table using a case-insensitive approach:
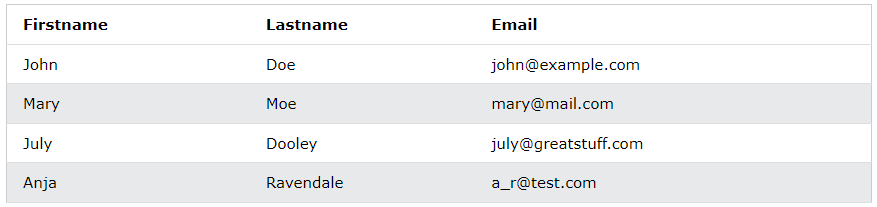
jQuery
<script>
$(document).ready(function(){
$("#myInput").on("keyup", function() {
var value = $(this).val().toLowerCase();
$("#myTable tr").filter(function() {
$(this).toggle($(this).text().toLowerCase().indexOf(value) > -1)
});
});
});
</script>
Explanation of the example: We loop through each table row using jQuery to see whether any text values match the input field value. The row (display:none) that does not match the search is hidden using the toggle() method. By converting the text to lower case using the toLowerCase() DOM API, we can make the search case insensitive, allowing “john,” “John,” and even “JOHN” to be found.
Filtering Item Lists
Search for items in a list without regard to case:
- First item
- Second item
- Third item
- Fourth
Filter Anything
Search for items in a list without regard to case:
I am a paragraph.
I am a div element inside div.