refers to the practice of embedding React components directly into an HTML file without using a build process or a separate JavaScript file. This is typically done by including the React library in a <script>
tag and then using JSX (JavaScript XML) directly within the HTML to render React components.
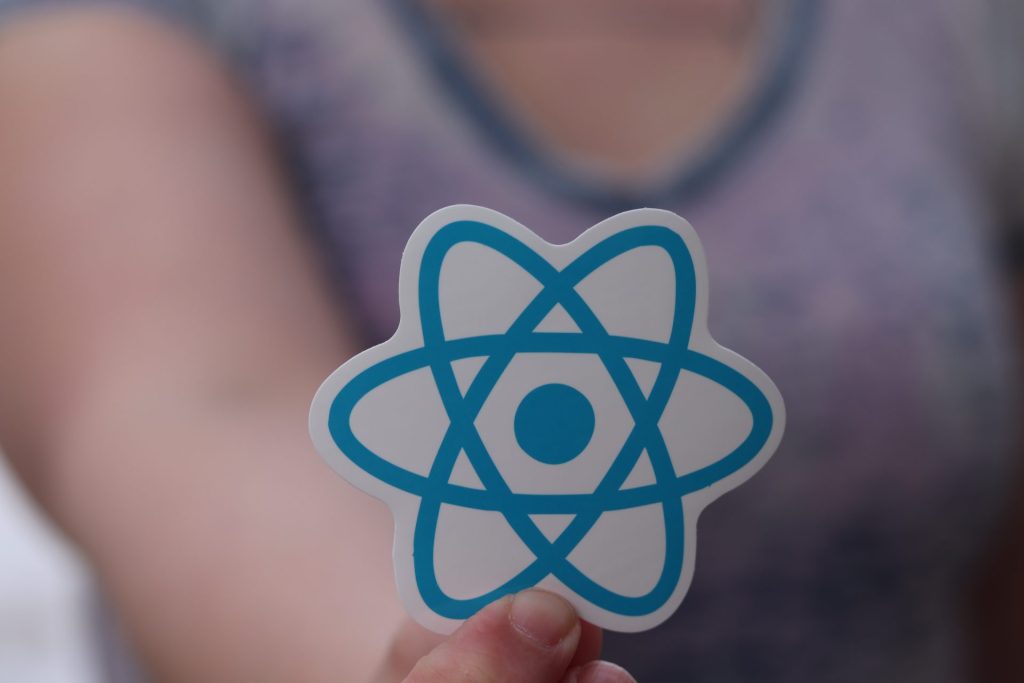
A rapid approach to learning React involves directly integrating React code within your HTML files.
Further information on JSX is provided in the chapter dedicated to that topic.
<!DOCTYPE html>
<html>
<head>
<script src="https://unpkg.com/react@18/umd/react.development.js" crossorigin></script>
<script src="https://unpkg.com/react-dom@18/umd/react-dom.development.js" crossorigin></script>
<script src="https://unpkg.com/@babel/standalone/babel.min.js"></script>
</head>
<body>
<div id="mydiv"></div>
<script type="text/babel">
function Hello() {
return <h1>Hello World!</h1>;
}
const container = document.getElementById('mydiv');
const root = ReactDOM.createRoot(container);
root.render(<Hello />)
</script>
</body>
</html>
This simple way of using React is fine for experimentation, but building a production-ready application necessitates setting up a complete React development environment.
Building a React Development Environment
To create a React app, make sure you have npx and Node.js installed, then use the create-react-app
command.
To guarantee that npx always uses the most recent version of create-react-app, it is advised that you delete the package if you have already installed it globally.
Use the following command to remove it: npm uninstall -g create-react-app.
npx create-react-app my-react-app
create-react-app
automates the configuration of all necessary components for the execution of a React application.
Deploying Your React Application
You are now prepared to launch your very first React application!
To get to the my-react-app directory, run this command:
cd my-react-app
To run the React application my-react-app
, execute this command:
npm start
Your freshly made React App will appear in a new browser window! Or else type localhost:3000 into the URL bar of your browser.
The outcome is:
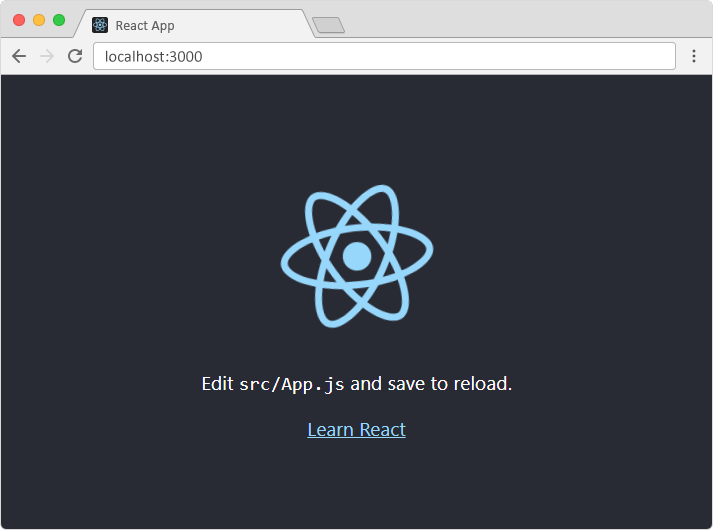
React Application Modification
Everything is going OK thus far, but how can I alter the content?
You may find the src folder in the my-react-app directory. When you open the App.js file located in the src folder, it will appear as follows:
/myReactApp/src/App.js:
import logo from './logo.svg';
import './App.css';
function App() {
return (
<div className="App">
<header className="App-header">
<img src={logo} className="App-logo" alt="logo" />
<p>
Edit <code>src/App.js</code> and save to reload.
</p>
<a
className="App-link"
href="https://reactjs.org"
target="_blank"
rel="noopener noreferrer"
>
Learn React
</a>
</header>
</div>
);
}
export default App;
Experiment by altering the HTML and saving the file.
You'll notice that you don't need to reload the browser to see the changes once you save the file!
Example
Put a <h1>
element in place of all th material inside the <div classname="App">
.
Click Save to view the changes in the browser.
function App() {
return (
<div className="App">
<h1>Hello World!</h1>
</div>
);
}
export default App;
You will see that we have eliminated the imports of logo.svg and app.css that we do not require.
You will see that we have eliminated the imports of logo.svg and app.css that we do not require.
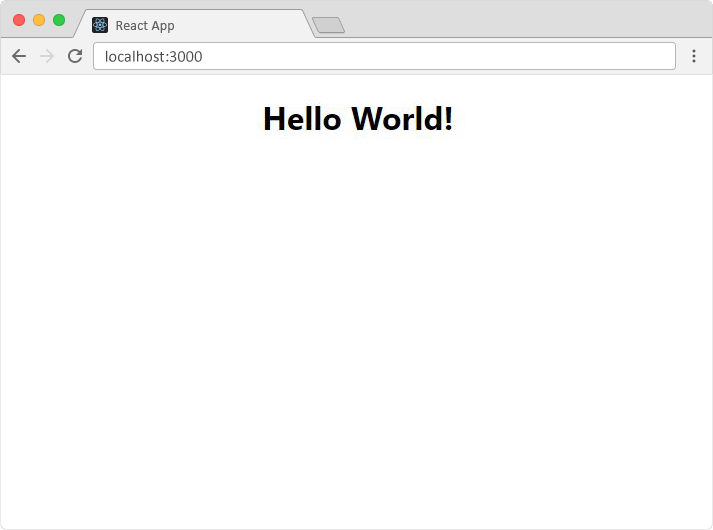
Installing React
To set up a React project, you’ll need to follow these steps:
Step 1: Install Node.js and npm (or yarn)
React relies on Node.js and npm (Node Package Manager), or optionally yarn, for installation and project management.
- Download and Install Node.js: Go to the official Node.js website (https://nodejs.org/) and download the installer for your operating system. The installer usually includes npm. Verify the installation by opening your terminal or command prompt and typing
node -v
andnpm -v
. You should see the versions printed. - (Optional) Install yarn: Yarn is an alternative package manager. If you prefer to use it, you can install it by following the instructions on the official Yarn website (https://yarnpkg.com/).
Step 2: Create a React Project using create-react-app
This is the easiest way to get started. create-react-app
sets up a basic React project with all the necessary configurations.
- Open your terminal or command prompt.
- Navigate to the directory where you want to create your project.
- Run the command:
npx create-react-app my-app
(Replacemy-app
with your desired project name). This will take a few minutes to complete. If you are using yarn, useyarn create react-app my-app
instead.
Step 3: Start the Development Server
- Navigate into your new project directory:
cd my-app
- Run the command:
npm start
(oryarn start
). This will start a development server and open your project in your web browser (usually athttp://localhost:3000
).
Step 4: Basic React Code Example (App.js)
Once the server is running, you’ll find the basic React code in the src/App.js
file. Here’s a slightly enhanced example:
import React from 'react';
import './App.css';
function App() {
const name = "World"; // Example variable
const date = new Date();
return (
<div className="App">
<h1>Hello, {name}!</h1>
<p>The current date and time is: {date.toLocaleString()}</p>
<MyComponent /> {/* Example of using a separate component */}
</div>
);
}
function MyComponent() {
return <p>This is a separate component!</p>;
}
export default App;
This code shows:
- Importing
React
(essential for React components). - Defining a functional component
App
. - JSX (JavaScript XML) syntax for creating UI elements. Note the curly braces
{}
for embedding JavaScript expressions within JSX. - A separate component
MyComponent
for demonstrating component reusability.
Step 5: Build for Production
When your app is ready, you can build it for production by running npm run build
(or yarn build
). This creates an optimized version of your app in the build
folder, ready for deployment.