A high-level Python web framework called Django makes it simple and quick for programmers to create online apps. Here is a quick guide on how to get Django up and running:
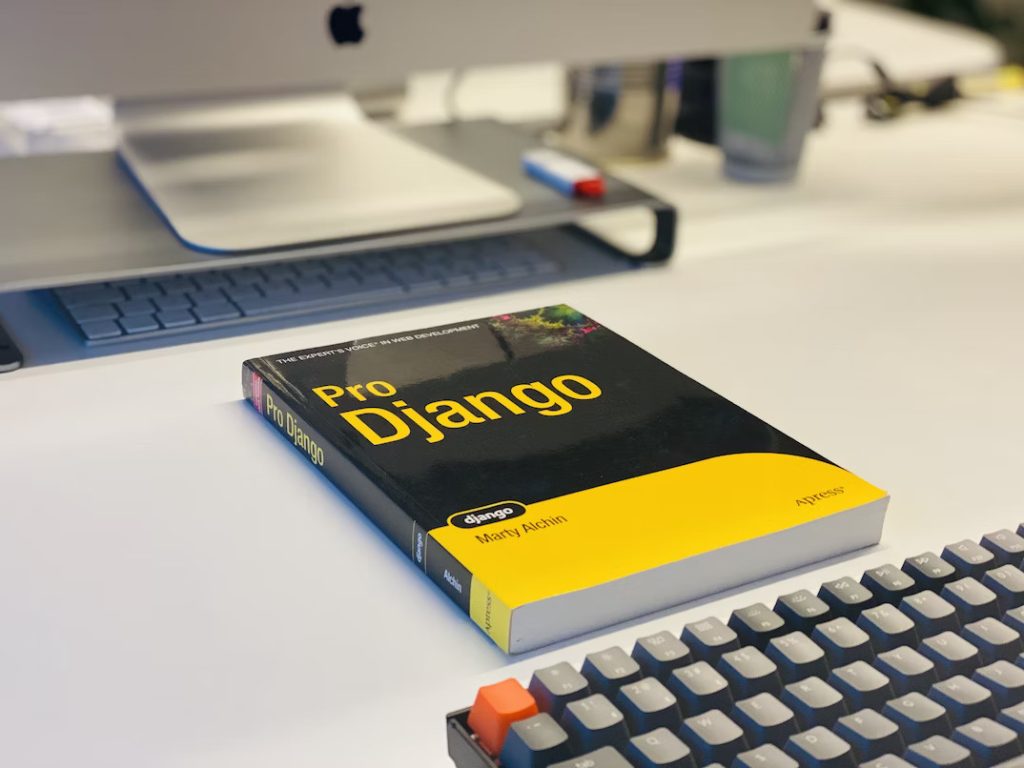
1. Install Django: To get started with Django, you need to have Python installed on your computer. You can then install Django using pip, the Python package manager, with the following command: pip install Django
2. New Django project creation is possible by using the django-admin command once Django has been installed. Run the command django-admin startproject myproject in a terminal or command prompt after opening the directory where you wish to build your project.
3. Create a new Django app: A project in Django is made up of one or more apps, each of which has a unique set of features. Navigate to your project directory and issue the command python manage.py startapp myapp to create a new app.
4. Define a model: A model is a Python class that represents a database table. In Django, you define your models using the django.db.models module. Here’s an example of a simple model that represents a blog post:
from django.db import models
class Post(models.Model):
title = models.CharField(max_length=200)
content = models.TextField()
created_at = models.DateTimeField(auto_now_add=True)
5. After you’ve created your model, you need to construct a database table that corresponds to it. Run the following commands in your terminal to accomplish this:
python manage.py makemigrations
python manage.py migrate
6. Create a view: A view is a Python function that handles a request from a client and returns a response. In Django, you define your views using the django.views module. Here’s an example of a simple view that displays a list of blog posts:
from django.shortcuts import render
from .models import Post
def post_list(request):
posts = Post.objects.all()
return render(request, 'myapp/post_list.html', {'posts': posts})
7. Create a template: A template is an HTML file that defines how your content will be rendered. In Django, you define your templates using the Django template language. Here’s an example of a simple template that displays a list of blog posts:
{% extends 'base.html' %}
{% block content %}
<h1>Blog Posts</h1>
<ul>
{% for post in posts %}
<li>{{ post.title }} - {{ post.created_at }}</li>
{% endfor %}
</ul>
{% endblock %}
8. Define a URL pattern: A URL pattern maps a URL to a view in your application. In Django, you define your URL patterns using the django.urls module. Here’s an example of a simple URL pattern that maps the URL /posts/ to our post_list view:
from django.urls import path
from .views import post_list
urlpatterns = [
path('posts/', post_list, name='post_list'),
]
9. Run your application: Finally, you can run your Django application using the following command: python manage.py runserver
And that’s it! You should now have a functioning Django application that displays a list of blog posts when you visit the URL http://localhost:8000/posts/.
Create Virtual Environment using Django
In Django, a virtual environment is a self-contained Python environment that allows you to isolate project dependencies and avoid conflicts with other Python projects. It ensures that the packages and libraries installed for one Django project do not interfere with another project.
To create a virtual environment in Django, you can follow these steps:
1. Activate the terminal or command prompt.
2. Change to the directory where you want to create your Django project.
3. To build a new virtual environment, use the following command:
python -m venv myenv
If you choose a different name for your virtual environment, substitute myenv.
4. The virtual environment should be turned on:
- On Windows:
myenv\Scripts\activate
- On macOS and Linux:
source myenv/bin/activate
The virtual environment name will appear on your command prompt or terminal after it has been started.
5. Installing the project’s dependencies, including Django:
pip install django
You can also install other packages required for your Django project using pip.
6. Now you can create your Django project using the django-admin command:
django-admin startproject myproject
Replace myproject with the desired name for your Django project.
7. The project directory should be changed:
cd myproject
8. You’re ready to start developing your Django project within the virtual environment.
To deactivate the virtual environment, simply use the deactivate command in your command prompt or terminal.
Creating a virtual environment helps keep your Django project’s dependencies organized and separate from the global Python environment, ensuring smoother development and deployment.
Project Django Creation
In Django, creating a project involves setting up the initial directory structure and configuration files for your web application. Here’s how you can create a Django project:
1. Activate the terminal or command prompt.
2. Go to the directory where your Django project is to be created.
3. To start a fresh Django project, use the command below:
django-admin startproject myproject
Change myproject to the name you’ve chosen for your project.
4. After running the command, Django will create a new directory with the specified project name (myproject in this example) containing the initial project structure and configuration files.
The directory structure of a Django project typically looks like this:
myproject/
manage.py
myproject/
__init__.py
settings.py
urls.py
asgi.py
wsgi.py
- The manage.py file is a command-line utility for managing the Django project. It provides various commands to run the development server, perform database migrations, and more.
- The Python package for your project is located within the myproject directory. It will be called just like your project.
- The directory is identified as a Python package by the __init__.py file.
- The settings.py file contains the project’s settings, including database configuration, installed apps, middleware, and other project-specific configurations.
- The URL patterns for your project are defined in the urls.py file.
- The entry points for ASGI and WSGI servers, respectively, are asgi.py and wsgi.py files. They take care of the correspondence between the web server and your Django application.
Once the project is created, you can start developing your Django application by creating Django apps within the project, defining models, views, templates, and configuring the URLs.
To run the development server and see your Django project in action, navigate to the project directory (myproject in this example) and run the following command:
python manage.py runserver
This will start the development server, and you can access your Django application in your web browser at http://localhost:8000/.
By creating a Django project, you establish the foundation for building a web application using Django’s powerful features and conventions.
Django Develop an App
In Django, the create app command is used to create a new Django application within a Django project. An application in Django is a self-contained module that houses a specific functionality of a web project. It can have its own models, views, templates, static files, and other components.
To create a new Django app, you can use the following command in the terminal or command prompt:
python manage.py startapp <app_name>
Here, <app_name> should be replaced with the desired name for your application. The command will create a directory with the given name and generate the necessary files and folders for your app.
Once the app is created, you need to include it in your Django project’s settings. Open the settings.py file in your project directory and locate the INSTALLED_APPS list. Add the name of your app to this list, like so:
INSTALLED_APPS = [
...
'<app_name>',
...
]
After creating the app, you can define models in the models.py file, views in the views.py file, and templates in the templates directory within your app’s directory. You can also define URL routing specific to your app by creating a urls.py file.
Here’s a simple example to demonstrate the usage of the create app command:
1. A command prompt or terminal should now be open.
2. Access the directory for your Django project.
3. Execute the next command:
python manage.py startapp polls
This will create a new app named polls.
4. ‘polls’ should be added to the INSTALLED_APPS list in the settings.py file of your project.
5. Make a model in the polls directory’s models.py file:
from django.db import models
class Question(models.Model):
question_text = models.CharField(max_length=200)
pub_date = models.DateTimeField('date published')
6. Under the polls directory, under the views.py file, create the following view:
from django.shortcuts import render
from django.http import HttpResponse
def index(request):
return HttpResponse("Hello, world. You're at the polls index.")
7. The following code should be added to the polls directory’s newly created urls.py file:
from django.urls import path
from . import views
app_name = 'polls'
urlpatterns = [
path('', views.index, name='index'),
]
8. Last but not least, start the development server using python manage.py runserver and open your browser to http://localhost:8000/polls/.
This is a basic example, but it should give you an idea of how to create an app in Django and define its components. You can further explore Django’s documentation to learn about more advanced features and concepts.
Views of Django
The Python methods or classes used in Django’s views manage the logic involved in processing a web request and producing a web response. They take user requests, connect with databases and models as needed, and render templates to provide the right answer.
Views are responsible for extracting data from the request, processing it, and determining what response to send back to the user. They can perform various tasks, such as fetching data from a database, performing calculations, rendering templates, and returning different types of responses like HTML, JSON, or redirects.
Here’s a breakdown of how views work in Django:
1. Function-Based Views:
Function-based views are defined as Python functions that take a request object as the first argument and return a response object. They are the simplest way to define views in Django. Here’s an example:
from django.http import HttpResponse
def hello(request):
return HttpResponse("Hello, World!")
In this example, the hello view takes a request object and returns an HTTP response with the message “Hello, World!”.
2. Class-Based Views:
Class-based views (CBVs) are another way to define views in Django. They are implemented as classes that inherit from Django’s View or its subclasses. CBVs provide a more object-oriented approach and often come with built-in functionality and mixins for common tasks. Here’s an example:
from django.views import View
from django.http import HttpResponse
class HelloView(View):
def get(self, request):
return HttpResponse("Hello, World!")
In this example, the HelloView class defines a get method that handles GET requests and returns an HTTP response with the message “Hello, World!”.
3. Rendering Templates:
Views often render templates to generate dynamic HTML responses. Django provides a rendering function called render that simplifies this process. The render function takes the request object, the template name, and a context dictionary (optional) as parameters. Here’s an example:
from django.shortcuts import render
def home(request):
context = {'name': 'John'}
return render(request, 'home.html', context)
In this example, the home view renders the home.html template with a context variable named name set to ‘John’. The rendered HTML response is then returned to the user.