For the v-slot directive to refer to named slots, it is necessary.
The placement of the information within the template of the child component can be more precisely controlled with named slots.
Components with named slots can be made more reusable and adaptable.
Prior to utilizing v-slots and named slots, let’s examine the consequences of utilizing two slots in the component.
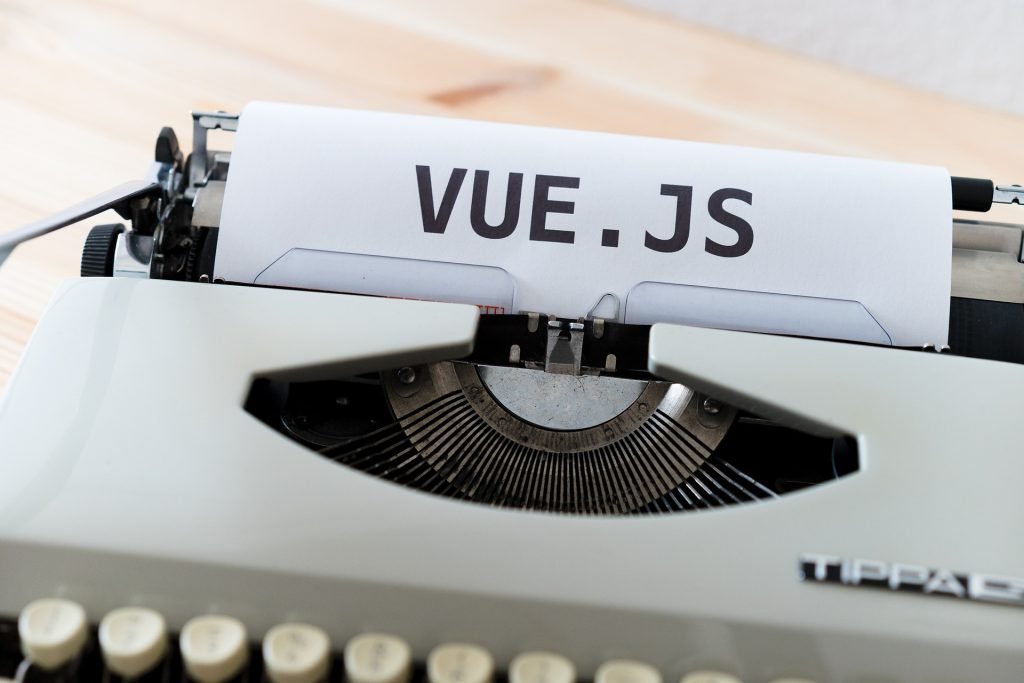
Example
App . vue :
<h1>App.vue</h1>
<p>The component has two div tags with one slot in each.</p>
<slot-comp>'Hello!'</slot-comp>
SlotComp . vue :
<h3>Component</h3>
<div>
<slot></slot>
</div>
<div>
<slot></slot>
</div>
We can observe that when a component has two slots, the material merely shows up in both locations.
Vue.js: v-slot and Named Slot Syntax
To control which a component’s content should appear in, identify the slots and use v-slot to send the content to the appropriate location.
Example
We give the slots distinct names so that we can distinguish between them.
SlotComp . vue :
<h3>Component</h3>
<div>
<slot name="topSlot"></slot>
</div>
<div>
<slot name="bottomSlot"></slot>
</div>
We can now direct the content to the appropriate slot by using App.vue’s v-slot.
App . vue :
<h1>App.vue</h1>
<p>The component has two div tags with one slot in each.</p>
<slot-comp v-slot:bottomSlot>'Hello!'</slot-comp>
Vue Default Slots
For components tagged with v-slot:default or those not marked with v-slot, a without a name will be used by default.
We only need to make two little adjustments to our earlier example to show how this works:
Example
SlotComp . vue :
<h3>Component</h3>
<div>
<slot name="topSlot"></slot>
</div>
<div>
<slot name="bottomSlot"></slot>
</div>
App . vue :
<h1>App.vue</h1>
<p>The component has two div tags with one slot in each.</p>
<slot-comp v-slot:bottomSlot>'Hello!'</slot-comp>
To further emphasize that the content is in the default slot, we can label it with the default value v-slot:default, as was previously described.
Example
SlotComp . vue :
<h3>Component</h3>
<div>
<slot></slot>
</div>
<div>
<slot name="bottomSlot"></slot>
</div>
App . vue :
<h1>App.vue</h1>
<p>The component has two div tags with one slot in each.</p>
<slot-comp v-slot:default>'Default slot'</slot-comp>
Vue v-slots in Template Tags
As you can see, the component tag can use the v-slot directive as an attribute.
Larger portions of text can also be sent to a specific by using v-slot in a
Example
App . vue :
<h1>App.vue</h1>
<p>The component has two div tags with one slot in each.</p>
<slot-comp>
<template v-slot:bottomSlot>
<h4>To the bottom slot!</h4>
<p>This p tag and the h4 tag above are directed to the bottom slot with the v-slot directive used on the template tag.</p>
</template>
<p>This goes into the default slot</p>
</slot-comp>
SlotComp . vue :
<h3>Component</h3>
<div>
<slot></slot>
</div>
<div>
<slot name="bottomSlot"></slot>
</div>
Since the element is merely a placeholder for the content and is not rendered, we utilize it to specify which should receive certain material. Examining the constructed page will reveal that the template tag is not there.
# Shorthand for v-slots
V-slot: can be shortened to #.
Thus, it follows:
<slot-comp v-slot:topSlot>'Hello!'</slot-comp>
This can be expressed as:
<slot-comp #topSlot>'Hello!'</slot-comp>
Example
App . vue :
<h1>App.vue</h1>
<p>The component has two div tags with one slot in each.</p>
<slot-comp #topSlot>'Hello!'</slot-comp>
SlotComp . vue :
<h3>Component</h3>
<div>
<slot name="topSlot"></slot>
</div>
<div>
<slot name="bottomSlot"></slot>
</div>
Vue.js Scoped Slot Syntax and Usage
A Scoped slot offers local data from the component, allowing the parent to choose how to render it.
Child-to-Parent Data Communication in Vue
We utilize v-bind in the component slot to communicate local data to the parent.
SlotComp.vue:
<template>
<slot v-bind:lclData="data"></slot>
</template>
<script>
export default {
data() {
return {
data: 'This is local data'
}
}
}
</script>
The data inside the component is referred to be ‘local’ since it is inaccessible to the parent until it is transmitted up to the parent, which we accomplish here with v-bind.
Accessing Data Passed from a Scoped Slot
The component’s local data is sent using v-bind and may be received in the parent using v-slot:
Example
App.vue:
<slot-comp v-slot:"dataFromSlot">
<h2>{{ dataFromSlot.lclData }}</h2>
</slot-comp>
In the prior example, ‘dataFromSlot’ is simply a name we could use to represent the data object we received from the scoped slot. We use the ‘lclData’ attribute to extract the text string from the slot and interpolate it into a <h2>
tag.
Scoped Slots & Arrays
A scoped slot can transport data from an array using v-for, however the code in App.vue is almost identical:
Example
SlotComp.vue:
<template>
<slot
v-for="x in foods"
:key="x"
:foodName="x"
></slot>
</template>
<script>
export default {
data() {
return {
foods: ['Apple','Pizza','Rice','Fish','Cake']
}
}
}
</script>
App.vue
:
<slot-comp v-slot="food">
<h2>{{ food.foodName }}</h2>
</slot-comp>
Using Scoped Slots to Display Arrays of Objects in Vue
A scoped slot can convey data from an array of objects using v-for.
Example
SlotComp.vue:
<template>
<slot
v-for="x in foods"
:key="x.name"
:foodName="x.name"
:foodDesc="x.desc"
:foodUrl="x.url"
></slot>
</template>
<script>
export default {
data() {
return {
foods: [
{ name: 'Apple', desc: 'Apples are a type of fruit that grow on trees.', url: 'img_apple.svg' },
{ name: 'Pizza', desc: 'Pizza has a bread base with tomato sauce, cheese, and toppings on top.', url: 'img_pizza.svg' },
{ name: 'Rice', desc: 'Rice is a type of grain that people like to eat.', url: 'img_rice.svg' },
{ name: 'Fish', desc: 'Fish is an animal that lives in water.', url: 'img_fish.svg' },
{ name: 'Cake', desc: 'Cake is something sweet that tastes good but is not considered healthy.', url: 'img_cake.svg' }
]
}
}
}
</script>
App.vue:
<slot-comp v-slot="food">
<hr>
<h2>{{ food.foodName }}<img :src=food.foodUrl></h2>
<p>{{ food.foodDesc }}</p>
</slot-comp>
Static Data in Scoped Slots
A scoped slot can also send static data, which is data that does not match the Vue instance’s data field.
When sending static data, we do not employ v-bind.
To demonstrate the difference, we transmit one static text and one text tied dynamically to the data instance.
Example
SlotComp.vue:
<template>
<slot
staticText="This text is static"
:dynamicText="text"
></slot>
</template>
<script>
export default {
data() {
return {
text: 'This text is from the data property'
}
}
}
</script>
App.vue:
<slot-comp v-slot="texts">
<h2>{{ texts.staticText }}</h2>
<p>{{ texts.dynamicText }}</p>
</slot-comp>
Vue Named Scoped Slots
Scoped slots can be assigned names.
To use named scoped slots, the slots within the component must be named with the ‘name’ attribute.
To receive data from a named slot, we must use the v-slot directive, or abbreviated #, in the parent component.
Example
In this example, the component is produced twice: once for the slot “leftSlot” and once for the slot “rightSlot“.
SlotComp.vue:
<template>
<slot
name="leftSlot"
:text="leftText"
></slot>
<slot
name="rightSlot"
:text="rightText"
></slot>
</template>
<script>
export default {
data() {
return {
leftText: 'This text belongs to the LEFT slot.',
rightText: 'This text belongs to the RIGHT slot.'
}
}
}
</script>
App.vue:
<slot-comp #leftSlot="leftProps">
<div>{{ leftProps.text }}</div>
</slot-comp>
<slot-comp #rightSlot="rightProps">
<div>{{ rightProps.text }}</div>
</slot-comp>
Alternatively, we can create the component once and use two separate “template” tags, each corresponding to a different slot.
Example
In this example, the component is built only once, but with two “template” tags, each referring to a distinct slot.
The code for SlotComp.vue is identical to that used in the previous example.
App.vue:
<slot-comp>
<template #leftSlot="leftProps">
<div>{{ leftProps.text }}</div>
</template>
<template #rightSlot="rightProps">
<div>{{ rightProps.text }}</div>
</template>
</slot-comp>