jQuery Effects are pre-defined animations and transitions that can be applied to HTML elements using the jQuery library. These effects provide a way to enhance the user experience on a website by adding visual appeal and interactivity.
Here’s an example of how to fade in an HTML element using a jQuery effect:
HTML:
<div id="myElement">
Hello, jQuery Effects!
</div>
JavaScript:
$(document).ready(function() {
$("#myElement").hide().fadeIn(1000); // Fades in the element over 1 second (1000 milliseconds)
});
In the example above, we use the hide() method to initially hide the element, and then we chain the fadeIn() method to gradually fade it in over a specified duration of 1000 milliseconds (1 second).
jQuery provides a wide range of effects, including sliding, fading, toggling, animating, and more. These effects can be combined, customized, and applied to different events such as button clicks or page load.
Hide and Show in jQuery
In jQuery, the hide() and show() methods are used to control the visibility of HTML elements on a web page.
The hide() method is used to hide an element by setting its CSS display property to “none”. This effectively removes the element from the visible layout of the page.
Here’s an example of using the hide() method:
HTML:
<div id="myElement">
This element will be hidden.
</div>
<button id="hideButton">Hide</button>
JavaScript:
$(document).ready(function() {
$("#hideButton").click(function() {
$("#myElement").hide();
});
});
In this example, we have an HTML element with the id “myElement” and a button with the id “hideButton”. When the button is clicked, the hide() method is called on the “myElement” element, making it disappear from the page.
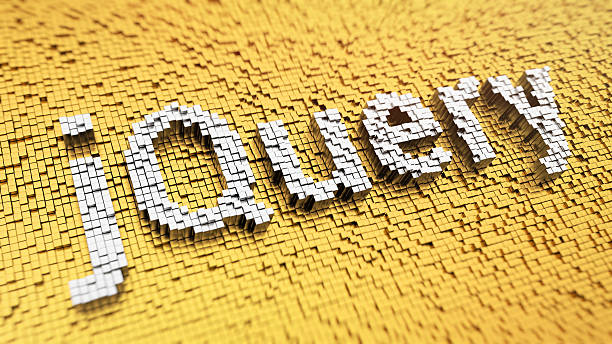
Here’s an example of using the show() method:
HTML:
<div id="myElement" style="display: none;">
This element is initially hidden.
</div>
<button id="showButton">Show</button>
JavaScript:
$(document).ready(function() {
$("#showButton").click(function() {
$("#myElement").show();
});
});
In this example, the “myElement” element is initially hidden using the inline style display: none;. When the button with the id “showButton” is clicked, the show() method is called on the “myElement” element, making it visible again.
Both the hidden() and show() functions allow a duration parameter to alter the animation pace. For example, you may use hide(1000) to progressively conceal the element over 1 second.
Fading using jQuery Effects
In jQuery, fading effects are a set of animations that gradually change the opacity of an element, creating smooth transitions between visibility states. The fading effects include fadeIn(), fadeOut(), fadeToggle(), and fadeTo().
Here’s a brief explanation of each fading effect along with an example:
fadeIn(): Fades in the selected element, gradually increasing its opacity until it becomes fully visible.
$(document).ready(function() {
$("#myElement").fadeIn(1000); // Fades in the element over 1 second (1000 milliseconds)
});
fadeOut(): Fades out the selected element, gradually decreasing its opacity until it becomes fully hidden.
$(document).ready(function() {
$("#myElement").fadeOut(1000); // Fades out the element over 1 second (1000 milliseconds)
});
fadeToggle(): Uses a fading effect to toggle the visibility of the chosen element. When an element is visible, it is faded out; when it is concealed, it is faded in.
$(document).ready(function() {
$("#myElement").fadeToggle(1000); // Toggles the visibility of the element over 1 second (1000 milliseconds)
});
fadeTo(): Changes the opacity of the selected element to a specific value. The first parameter is the duration, and the second parameter is the target opacity value (ranging from 0 to 1).
$(document).ready(function() {
$("#myElement").fadeTo(1000, 0.5); // Changes the opacity of the element to 0.5 over 1 second (1000 milliseconds)
});
In these examples, #myElement refers to the HTML element you want to apply the fading effect to. The duration parameter specifies the time it takes for the fading animation to complete, given in milliseconds. Adjust the duration value to control the speed of the fade.
Sliding using jQuery Effects
In jQuery, sliding effects are animations that change the visibility and position of elements by sliding them in or out of view. The sliding effects include slideUp(), slideDown(), and slideToggle().
Here’s a brief explanation of each sliding effect along with an example:
slideUp(): Slides up (hides) the selected element by animating its height to 0.
$(document).ready(function() {
$("#myElement").slideUp(1000); // Slides up the element over 1 second (1000 milliseconds)
});
slideDown(): Slides down (reveals) the selected element by animating its height from 0 to its original height.
$(document).ready(function() {
$("#myElement").slideDown(1000); // Slides down the element over 1 second (1000 milliseconds)
});
slideToggle(): Uses a sliding effect to change the visibility of the chosen element. The element will be slid up if it is visible and down if it is hidden.
$(document).ready(function() {
$("#myElement").slideToggle(1000); // Toggles the visibility of the element over 1 second (1000 milliseconds)
});
The HTML element you wish to use for the sliding effect is identified in these instances as #myElement. The duration parameter, which is specified in milliseconds, indicates how long it takes for the sliding animation to finish. To alter the slide’s pace, change the time parameter.
Note that sliding effects manipulate the height property of the element. If you want to slide an element horizontally, you can use CSS properties like width, marginLeft, or marginRight and apply similar jQuery effects (animate() method) to achieve the desired sliding animation.
Remember to include the jQuery library before using these sliding effects.
Animation with jQuery Effects
In jQuery, animation effects provide a way to create custom and complex animations by manipulating CSS properties of HTML elements over time. The primary method used for animation is the animate() method.
Here’s a general definition and an example of using the animate() method for creating animations in jQuery:
Definition:
The animate() method in jQuery allows you to animate the CSS properties of an element by specifying the target values and the duration of the animation.
Example:
Let’s consider an example where we animate the width and opacity of an
element:
HTML:
<div id="myElement"></div>
CSS:
#myElement {
background-color: blue;
width: 100px;
height: 100px;
}
JavaScript:
$(document).ready(function() {
$("#myElement").animate({
width: "300px",
opacity: 0.5
}, 1000); // Animates the width and opacity over 1 second (1000 milliseconds)
});
In this example, we use its ID to pick the myElement element and then run the animation() function on it. We supply an object with the CSS attributes we want to animate (width and opacity) and their associated goal values (“300px” and 0.5) within the animation() function. Finally, we specify 1000 milliseconds (1 second) for the duration of the animation.
During the animation, the width of the element will gradually increase from its initial value of 100 pixels to 300 pixels, and the opacity will change from its initial value of 1 to 0.5, creating a smooth transition.
You can animate multiple properties simultaneously and even include additional parameters like easing functions or callback functions for more control over the animation.
Before utilizing the animate() function or any other animation-related features, be sure you include the jQuery library.
Stop Animations in jQuery
The stop() function in jQuery is used to stop animations that are presently executing on specified items. It allows you to pause running animations and, if desired, delete the animation queue.
Here’s the definition and an example of using the stop() method in jQuery: Here’s the definition and an example of using the stop() method in jQuery:
Definition:
Animations on specific elements may be stopped using jQuery’s stop() method.
Example:
Assume we have a <div> element with an ongoing animation that expands its width, and we want the animation to halt when a button is clicked:
HTML:
<div id="myElement"></div>
<button id="stopButton">Stop Animation</button>
CSS:
#myElement {
background-color: blue;
width: 100px;
height: 100px;
}
JavaScript:
$(document).ready(function() {
$("#stopButton").click(function() {
$("#myElement").stop();
});
$("#myElement").animate({
width: "300px"
}, 3000); // Animates the width over 3 seconds
});
In this example, we have a button with the ID “stopButton” that triggers the animation stop. When the button is clicked, the click() event handler function is executed. Inside the function, we use the stop() method on the “myElement” element to stop any ongoing animation on it.
Additionally, we have an animation on the “myElement” element using the animate() method. This animation increases the width of the element over 3 seconds.
When the button is clicked, the stop() method is called, and the ongoing animation is halted. The element will retain its current width, and any queued animations will be cleared.
You can also use the stop() method with parameters to clear the animation queue or jump to the end of the current animation. Refer to the jQuery documentation for more details on the different options available with the stop() method.
Remember to include the jQuery library before using the stop() method or any other jQuery functionality.
Callback Functions in jQuery
A callback function in jQuery is a function that is supplied as an input to another function and is performed after the main function has finished its purpose. Callback functions are frequently used in asynchronous processes or when you wish to do certain actions after an event or animation has completed.
Here’s a definition and an example of using callback functions in jQuery:
Definition:
A callback function in jQuery is a function that is passed as input to another function and is executed once the job of the main function is finished or when a certain event occurs.
Example: Let’s consider an example where we use a callback function to display an alert message after an animation has finished:
HTML:
<div id="myElement"></div>
<button id="animateButton">Animate</button>
CSS:
#myElement {
background-color: blue;
width: 100px;
height: 100px;
}
JavaScript:
$(document).ready(function() {
$("#animateButton").click(function() {
$("#myElement").animate({
width: "300px"
}, 1000, function() {
alert("Animation finished!");
});
});
});
In this example, we have a button with the ID “animateButton” that triggers an animation. When the button is clicked, the click() event handler function is executed. Inside the function, we use the animate() method on the “myElement” element to animate its width.
The animation() method’s third parameter is a callback function, which is specified inline as an anonymous function. When the animation is finished, this callback function will be called. In this situation, an alert message with the words “Animation finished!” is displayed.
When the button is clicked, the element’s width will animate from its initial value of 100 pixels to 300 pixels over a duration of 1000 milliseconds (1 second). Once the animation is complete, the callback function is invoked, and the alert message is displayed.
You can use callback functions in various scenarios, such as handling AJAX requests, animations, event listeners, or any asynchronous operation where you need to perform specific actions after a task is finished or an event occurs.
Remember to include the jQuery library before using callback functions or any other jQuery features.
Chaining with jQuery
In jQuery, chaining refers to the practice of chaining multiple calls together on the same set of elements. By chaining methods, you can apply a series of operations or modifications to an element or set of elements in a concise and readable manner.
Here is a description of method chaining in jQuery along with an demonstration:
Definition:
Chaining in jQuery allows you to call multiple methods on the same jQuery object or set of elements in a single line, with each method being called one after another.
Example:
Let’s say we have an <div> element that we want to modify by applying multiple jQuery methods in a chain:
HTML:
<div id="myElement">Hello, jQuery Chaining!</div>
JavaScript:
$(document).ready(function() {
$("#myElement")
.css("color", "blue")
.slideUp(1000)
.delay(2000)
.fadeIn(1000);
});
In this example, we select the element with the ID “myElement” using the $() function (or jQuery()) and start chaining methods on it.
The first method in the chain is css(), which sets the CSS property color to “blue” for the selected element. The next method is slideUp(), which slides up the element to hide it over a duration of 1000 milliseconds (1 second).
After that, we use the delay() method to introduce a pause of 2000 milliseconds (2 seconds) in the chain. Finally, we call the fadeIn() method to fade in the element over a duration of 1000 milliseconds (1 second) after the delay.
By chaining these methods together, we apply the CSS modification, slide up animation, delay, and fade in animation in a single line of code, making it more concise and readable.
Chaining can be a powerful technique in jQuery, allowing you to perform a sequence of operations on elements without having to repeatedly select or reference them.
Remember to include the jQuery library before using method chaining or any other jQuery features.