In Ruby, variables are used to store and manipulate data. They are dynamically typed, which means you don’t need to declare their type explicitly. Ruby variables start with a lowercase letter or an underscore (_).
Constants, on the other hand, are similar to variables, but their value cannot be changed once assigned. They are typically used for values that are not expected to change during the execution of a program. Ruby constants start with an uppercase letter.
Literals, in Ruby, are fixed values that are directly written into the code. They represent themselves and do not need to be stored in variables or constants.
Let’s see some examples:
Variables:
# Integer variable
age = 25
# String variable
name = "John"
# Floating-point variable
salary = 2500.50
# Boolean variable
is_student = true
Constants:
# Constant representing the value of pi
PI = 3.14159
# Constant representing the maximum number of attempts
MAX_ATTEMPTS = 3
Literals:
# Integer literal
count = 10
# String literal
message = "Hello, world!"
# Floating-point literal
pi = 3.14
# Boolean literal
is_valid = false
In the examples above, age, name, salary, and is_student are variables. PI and MAX_ATTEMPTS are constants. And count, message, pi, and is_valid are literals.
It’s worth mentioning that Ruby also has special variables and predefined constants. Special variables, such as $stdin and $stdout, are built-in variables with specific purposes. Predefined constants, such as nil, true, and false, represent special values in Ruby.
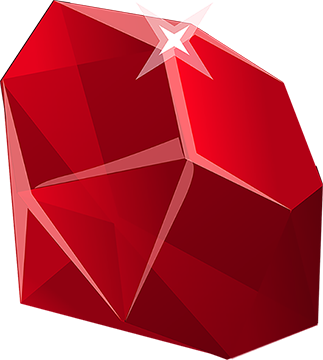
Operators in Ruby
In Ruby, operators are symbols or words that perform operations on operands (values or variables). There are several types of operators in Ruby, including arithmetic operators, assignment operators, comparison operators, logical operators, and more.
Let’s go through some of the commonly used operators in Ruby along with examples:
Arithmetic Operators:
# Addition
result = 10 + 5 # 15
# Subtraction
result = 10 - 5 # 5
# Multiplication
result = 10 * 5 # 50
# Division
result = 10 / 5 # 2
# Modulus (remainder)
result = 10 % 3 # 1
# Exponentiation
result = 2**3 # 8
Assignment Operators:
# Simple assignment
x = 10
# Addition assignment
x += 5 # Equivalent to x = x + 5
# Subtraction assignment
x -= 3 # Equivalent to x = x - 3
# Multiplication assignment
x *= 2 # Equivalent to x = x * 2
# Division assignment
x /= 4 # Equivalent to x = x / 4
# Modulus assignment
x %= 3 # Equivalent to x = x % 3
Comparison Operators:
# Equal to
result = 10 == 5 # false
# Not equal to
result = 10 != 5 # true
# Greater than
result = 10 > 5 # true
# Less than
result = 10 < 5 # false
# Greater than or equal to
result = 10 >= 5 # true
# Less than or equal to
result = 10 <= 5 # false
Logical Operators:
# Logical AND
result = true && false # false
# Logical OR
result = true || false # true
# Logical NOT
result = !true # false
These are just a few examples of the operators available in Ruby. Ruby also has bitwise operators, ternary operator, range operators, and more.
Comments in Ruby
In Ruby, comments are used to include illustrative or descriptive language that the interpreter ignores. They are helpful for describing the functioning of the code, explaining the code, or momentarily turning off code snippets. Ruby allows single-line and multi-line comments of both lengths.
Hash (#) marks the beginning of a single-line comment, which continues until the end of the line. Here’s an demonstration:
# This is a single-line comment in Ruby
puts "Hello, world!" # This line prints a greeting
In the above example, the first line is a comment, while the second line is an executable code statement.
Block comments are multi-line comments that begin with =begin and end with =end. Between these two marks, all lines are regarded as comments. Here’s a demonstration:
=begin
This is a multi-line comment in Ruby.
It can span across multiple lines.
=end
puts "This is the main code block."
In the above example, everything between =begin and =end is a comment, and the puts
statement is the executable code.
Comments are helpful for enhancing code readability, providing explanations, and making the code more maintainable. They are also useful for temporarily disabling code during development or troubleshooting by commenting it out.
It’s good practice to include comments that explain the code’s purpose, algorithms, complex logic, or any important information that future developers may need to understand.
If…else, case, unless in Ruby
Conditional statements in Ruby let you direct the course of execution depending on certain circumstances. If…else, case, and unless are three conditional constructs that are often used in Ruby. Let’s examine each one using the following instances:
1. if…else:
You may run various pieces of code using the if…else statement depending on a circumstance.
if condition
# code to be executed if the condition is true
else
# code to be executed if the condition is false
end
Here’s an example:
age = 18
if age >= 18
puts "You are eligible to vote."
else
puts "You are not eligible to vote yet."
end
2. case:
The case statement provides a way to check multiple conditions and execute different blocks of code based on the value of a variable or expression.
case expression
when value1
# code to be executed if expression matches value1
when value2
# code to be executed if expression matches value2
else
# code to be executed if expression doesn't match any value
end
Here’s an example:
day = "Monday"
case day
when "Monday"
puts "It's the beginning of the week."
when "Friday"
puts "It's the end of the week."
else
puts "It's a regular day."
end
3. unless:
The inverse of an if statement is a unless statement. You can only run a piece of code if a condition is false, according to this feature.
unless condition
# code to be executed if the condition is false
else
# code to be executed if the condition is true
end
Here’s an example:
grade = 85
unless grade >= 60
puts "You failed the exam."
else
puts "You passed the exam."
end
In the example above, if the condition grade >= 60 is false, the code within the unless block will be executed.
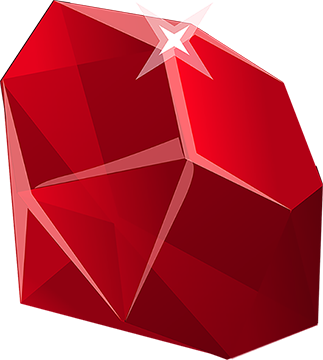
These conditional constructs provide different ways to control the flow of your Ruby code based on specific conditions. You can choose the one that best suits your needs and makes your code more readable and maintainable.
Loops in Ruby
Loops are used to continually run a piece of code in Ruby up until a certain condition is fulfilled. Ruby supports a number of loop types, including while, till, for, and each. Let’s examine each one using the following instances:
1. while loop:
As long as a specified condition is true, the while loop runs a block of code.
while condition
# code to be executed
end
Here’s an example:
counter = 0
while counter < 5
puts "Counter: #{counter}"
counter += 1
end
This loop will print the value of counter from 0 to 4.
2. until loop:
The until loop performs a block of code until a specified condition is met.
until condition
# code to be executed
end
Here’s an example:
counter = 0
until counter >= 5
puts "Counter: #{counter}"
counter += 1
end
This loop will also print the value of counter from 0 to 4.
3. for loop:
The for loop iterates over a range, array, or other enumerable objects.
for variable in collection
# code to be executed
end
Here’s an example:
for i in 1..5
puts "Number: #{i}"
end
The range of integers printed by this loop is 1 to 5.
4. each loop:
The each loop is used to iterate over elements in an array, hash, or other enumerable objects.
collection.each do |variable|
# code to be executed for each element
end
Here’s an example:
fruits = ["apple", "banana", "orange"]
fruits.each do |fruit|
puts "I like #{fruit}"
end
This loop will print “I like apple”, “I like banana”, and “I like orange”.
In addition to these loops, Ruby also provides other constructs like loop
and do…while loops. These loops give you additional flexibility and control over the flow of your code.
Remember to be cautious while using loops to avoid infinite loops. Make sure to include a condition or break statement to exit the loop when necessary.