A method in Ruby is a reusable section of code that completes a certain purpose. You may reuse your code throughout your application by grouping it into logical pieces. Methods can return values and take arguments (inputs).
Here’s the syntax for defining a method in Ruby:
def method_name(parameter1, parameter2, ...)
# Code block
# Perform task
# Optionally, return a value
end
Let’s examine each part of a technique individually:
- The phrase for defining a method is def.
- The method is identified by its name, method_name. Pick a name that accurately describes the method’s goal.
- The optional arguments or parameters that the method can accept are parameter1, parameter2, etc. You can use commas to separate zero or more arguments.
- The code block between do and end contains the instructions or logic of the method. It specifies the task the method performs.
- Optionally, the method can return a value using the return keyword.
Here’s an example of a method in Ruby:
def greet(name)
puts "Hello, #{name}!"
end
greet("John")
In this example, we define a method called greet that takes one parameter name
. The method prints a greeting message using the parameter value. When we call the method with the argument “John”, it outputs “Hello, John!” to the console.
You can also define methods that return values. Here’s an example:
def square(number)
return number * number
end
result = square(5)
puts result
In this example, we define a method called square that takes one parameter number. The method calculates the square of the input number and returns the result using the return keyword. When we call the method with the argument 5, it returns 25, which is assigned to the result variable. Finally, we print the result using puts.
Methods in Ruby are powerful constructs that allow you to encapsulate logic and reuse code throughout your program. They enhance code organization, readability, and maintainability.
Blocks in Ruby
Blocks are reusable sections of code in the Ruby programming language. They provide as a mechanism to package behavior and give it to other methods as an argument. Ruby’s blocks are a strong feature that are frequently used for callbacks, iteration, and modifying method behavior.
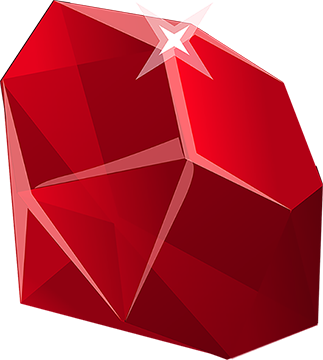
Blocks are defined using either the curly brace syntax {} or the do…end syntax. Here’s an example of a block defined using both syntaxes:
# Using curly braces
3.times { puts "Hello, world!" }
# Using do...end
3.times do
puts "Hello, world!"
end
In the above example, the times method is called on the number 3
, and a block is passed to it. The block contains the code puts “Hello, world!”, which is executed three times.
Parameters can also be accepted by blocks. At the start of the block, there are vertical bars with the word |
that specify the parameters. Here’s an demonstration:
# Using curly braces
[1, 2, 3].each { |num| puts num }
# Using do...end
[1, 2, 3].each do |num|
puts num
end
In this case, the each method is called on an array [1, 2, 3], and a block is passed to it. The block accepts a parameter num, which represents each element of the array. The code within the block puts num is executed for each element in the array.
Blocks can also be assigned to variables and executed later. This is useful for creating reusable blocks of code. Here’s an example:
# Block assigned to a variable
my_block = Proc.new { |name| puts "Hello, #{name}!" }
my_block.call("Alice") # Output: Hello, Alice!
my_block.call("Bob") # Output: Hello, Bob!
In this example, a block is assigned to the variable my_block using the Proc.new method. The block accepts a parameter name and prints a greeting. The call method is used to execute the block, passing different names as arguments.
Blocks are a fundamental concept in Ruby and are widely used throughout the language. They provide a flexible way to define custom behavior and make Ruby code more expressive and concise.
Modules and Mixins in Ruby
In Ruby, modules are a way to group related methods, constants, and classes together, allowing them to be reused in multiple places. Modules serve as containers for these definitions and provide a namespace to avoid conflicts with other parts of the code. Additionally, Ruby modules can be used to implement mixins, which allow classes to inherit behavior from multiple sources.
Here’s an example of defining a module in Ruby:
module MyModule
def greet
puts "Hello!"
end
end
In this example, we define a module called MyModule that contains a single method greet. Modules can have any number of methods and constants. To use the methods defined in a module, we can either include or extend the module in a class.
Including a module adds the module’s methods as instance methods of the class. Here’s an example:
class MyClass
include MyModule
end
obj = MyClass.new
obj.greet # Output: Hello!
In this case, the MyClass class includes the MyModule module using the include keyword. As a result, instances of MyClass gain access to the greet method defined in MyModule.
Extending a module adds the module’s methods as class methods of the class. Here’s an example:
class MyClass
extend MyModule
end
MyClass.greet # Output: Hello!
In this example, the MyClass class extends the MyModule module using the extend keyword. This allows the greet method to be called directly on the class itself.
Mixins are a powerful feature of Ruby that allows classes to inherit behavior from multiple modules. This is achieved by using both include and extend with multiple modules. Here’s an example:
module Module1
def method1
puts "Method 1"
end
end
module Module2
def method2
puts "Method 2"
end
end
class MyClass
include Module1
extend Module2
end
obj = MyClass.new
obj.method1 # Output: Method 1
MyClass.method2 # Output: Method 2
In this example, the MyClass class includes Module1 and extends Module2. As a result, instances of MyClass gain access to the method1, and the class itself gains access to method2.
Modules and mixins are essential tools in Ruby for organizing and reusing code. They enable better code organization, prevent naming conflicts, and provide a way to extend the functionality of classes.
Strings in Ruby
Strings are used to represent character sequences in Ruby. They are surrounded by either single quotes (‘) or double quotes (“). Here’s an example of how to define strings in Ruby:
single_quoted_string = 'Hello, world!'
double_quoted_string = "Hello, Ruby!"
puts single_quoted_string
puts double_quoted_string
Output:
Hello, world!
Hello, Ruby!
In the example above, we define two strings: single_quoted_string and double_quoted_string. The first one is defined using single quotes, and the second one is defined using double quotes. Both strings contain a greeting message.
You can use either single or double quotes to define strings in Ruby, but there are some differences between them. When you use single quotes, the string is treated literally, and escape sequences (like \n for newline) and variable interpolation (replacing placeholders with variable values) are not processed. On the other hand, when you use double quotes, escape sequences and variable interpolation are processed within the string.
Here’s an example that demonstrates variable interpolation:
name = "Alice"
age = 25
greeting = "Hello, my name is #{name} and I am #{age} years old."
puts greeting
Output:
Hello, my name is Alice and I am 25 years old.
In the above example, the string greeting includes placeholders (#{name} and #{age}) that are replaced with the values of the variables name and age respectively using variable interpolation.
Strings in Ruby are mutable, which means they can be modified. Strings can be concatenated by using the + operator or the technique. Here’s an example:
first_name = "John"
last_name = "Doe"
full_name = first_name + " " + last_name
full_name << " Jr."
puts full_name
Output:
John Doe Jr.
In the above example, we concatenate the first_name, a space, and the last_name to create the full_name string. Then, we append ” Jr.” to the full_name string using the << method.
Arrays in Ruby
An arrangement of characters known as a string is frequently used to represent text. Strings are a prominent data type in programming and are frequently used to store and work with textual data.
Examples:
1. Basic String Operations:
name = "Alice"
greeting = "Hello, " + name + "!"
puts greeting
Output:
Hello, Alice!
By joining the strings “Hello,” “, the value of the name variable, and “!” in this example, the string variable greeting is produced. Following that, the generated string is displayed to the console.
2. String Interpolation:
age = 25
message = "I am #{age} years old."
puts message
Output:
I am 25 years old.
Here, the value of the age variable is interpolated into the string using the #{} syntax. The placeholder #{age} is replaced with the value of the variable when the string is evaluated.
3. String Length and Indexing:
text = "Hello, World!"
length = text.length
first_character = text[0]
last_character = text[-1]
puts length
puts first_character
puts last_character
Output:
13
H
!
In this example, the string variable text is defined, and we use the length method to determine its length. We also use indexing to access specific characters within the string. The first character is obtained using index 0, and the last character is obtained using index -1.
4. String Methods:
sentence = "Ruby is a great programming language."
uppercase_sentence = sentence.upcase
reversed_sentence = sentence.reverse
puts uppercase_sentence
puts reversed_sentence
Output:
In this example, we define the string variable sentence and use the upcase method to convert it to uppercase. We also use the reverse method to reverse the order of characters in the string.