Dynamic, object-oriented programming language Ruby is renowned for being straightforward and readable. It was created with the goal of being programmer-friendly, with an emphasis on developer satisfaction and productivity. Here are some fundamental Ruby ideas and demonstrations:
1. Variables:
In Ruby, variables are denoted by an underscore or a lowercase letter. They may store a variety of values, including objects, strings, and integers.
Example:
name = "John"
age = 25
2. Strings:
Character groups that are separated by single or double quotes are called strings. Ruby offers a number of ways to alter and use strings.
Example:
greeting = "Hello, World!"
puts greeting
3. Arrays:
In Ruby, arrays are arranged groups of things. They are indexed from 0 and can hold various data types.
Example:
numbers = [1, 2, 3, 4, 5]
puts numbers[0] # Output: 1
4. Hashes:
Key-value pairs are stored in hashes, which are sometimes called dictionaries or associative arrays. All types of keys are distinct and can be used.
Example:
person = { "name" => "Alice", "age" => 30 }
puts person["name"] # Output: Alice
5. Conditionals:
Ruby provides if-else statements for conditional branching. It allows you to execute different code blocks based on certain conditions.
Example:
6. Loops:
Ruby offers different loop constructs like while, for, and each to iterate over collections or repeat code blocks.
Example:
numbers = [1, 2, 3, 4, 5]
numbers.each do |num|
puts num
end
These are just a few basic concepts in Ruby. The language has many more features and capabilities that make it powerful and flexible for a wide range of programming tasks.
Learning Ruby
Ruby is a high-level, interpreted programming language that was initially introduced in 1995 by Yukihiro Matsumoto, popularly known as “Matz.” Ruby is renowned for its brevity, readability, and productivity. It is an open-source programming language, which entails that anybody may download, modify, and share it for free.
Installation
You must first install Ruby on your computer. The official Ruby website is available at https://www.ruby-lang.org/en/downloads/. Pay attention to the instructions for your operating system.
Open a terminal or command prompt after installation, then execute ruby -v. It should show the version of Ruby that is currently installed on your computer if the installation was successful.
Running Ruby code
You can run Ruby code using a Ruby interpreter. Open a text editor, create a new file, and save it with a .rb extension. For example, hello.rb.
In the file, write the following code:
puts "Hello, World!"
Save the file and open a terminal or command prompt in the same directory where you saved the file. Type ruby hello.rb and hit enter. You should see the output Hello, World! on the terminal.
Basic Syntax
Comments
The # sign may be used to insert comments into your code. Anything typed after the hash mark (#) is regarded as a comment and won’t be carried out by the interpreter.
# This is a comment
puts "Hello, World!" # This is another comment
Variables
In Ruby, variables can be created using the following syntax:
variable_name = value
For example:
name = "John"
age = 25
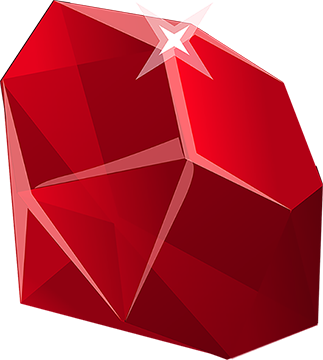
Data Types
Ruby includes various data types by default, including:
- Integers: whole numbers, e.g., 1, 2, 3, etc.
- Floats: decimal numbers, e.g., 1.5, 2.25, etc.
- Strings are character sequences such as “hello”, “world”, and so on.
- Booleans: true or false values
- Arrays: ordered lists of items, e.g., [1, 2, 3], [“apple”, “banana”, “orange”], etc.
- Hashes: collections of key-value pairs, e.g., { “name” => “John”, “age” => 25 }, etc.
Operators
Ruby has several operators that you can use to perform operations on variables and values, including:
- Arithmetic operators: +, –, *, /, %
- Comparison operators: ==, !=, <, >, <=, >=
- Logical operators: &&, ||, !
Conditional Statements
You can use conditional statements in Ruby to perform different actions based on different conditions. The basic syntax for a conditional statement is:
if condition
# code to execute if condition is true
else
# code to execute if condition is false
end
For example:
age = 25
if age > 18
puts "You are an adult"
else
puts "You are a minor"
end
Loops
In Ruby, loops may be used to execute a piece of code repeatedly. In Ruby, there are various forms of loops, including:
- while loop
- until loop
- for loop
- each loop
For example:
i = 0
while i < 5 do
puts i
i += 1
end
Methods
In Ruby, you may define your own methods using the following syntax:
def method_name(arguments)
# code to execute
end
For example:
def greet(name)
puts "Hello, #{name}!"
end
Classes and Objects in Ruby
Classes and objects are the core ideas of object-oriented programming in Ruby. An object’s structure and behavior are specified by a class, which functions as a blueprint or template. In contrast, an object is a tangible representation of a class that may retain data and carry out operations.
Here’s a definition and examples of classes and objects in Ruby:
1. Classes:
A class in Ruby encapsulates data and methods into a single entity. It defines the attributes and behavior that objects of that class will have.
Example:
class Person
attr_accessor :name, :age
def initialize(name, age)
@name = name
@age = age
end
def say_hello
puts "Hello, #{@name}!"
end
end
In the above example, the Person class has two attributes (name and age) and two methods (initialize and say_hello). The initialize method is a special method called a constructor, which is called when a new object is created. The say_hello method prints a greeting using the person’s name.
2. Objects:
Class instances are what make up objects. They stand for particular occurrences or people who are capable of carrying out the class’s prescribed activities and have their own special data.
Example:
person1 = Person.new("Alice", 30)
person2 = Person.new("Bob", 25)
puts person1.name # Output: Alice
puts person2.age # Output: 25
person1.say_hello # Output: Hello, Alice!
person2.say_hello # Output: Hello, Bob!
The objects produced from the Person class in the example above are person1 and person2. Each object has a unique set of properties (such as name and age) and is capable of using the class’s stated methods (such as say_hello).
By using classes and objects, you can create reusable and organized code structures in Ruby. Classes define the structure and behavior, while objects provide specific instances that can be manipulated and interacted with in your programs.