In AngularJS, tables can be easily created and manipulated using the built-in directives such as ng-repeat. Here’s an example of how to create a table using AngularJS:
<table>
<thead>
<tr>
<th>Name</th>
<th>Email</th>
</tr>
</thead>
<tbody>
<tr ng-repeat="user in users">
<td>{{ user.name }}</td>
<td>{{ user.email }}</td>
</tr>
</tbody>
</table>
In the above example, we create a basic HTML table with two columns (Name and Email) in the header row (thead) and an empty body row (tbody).
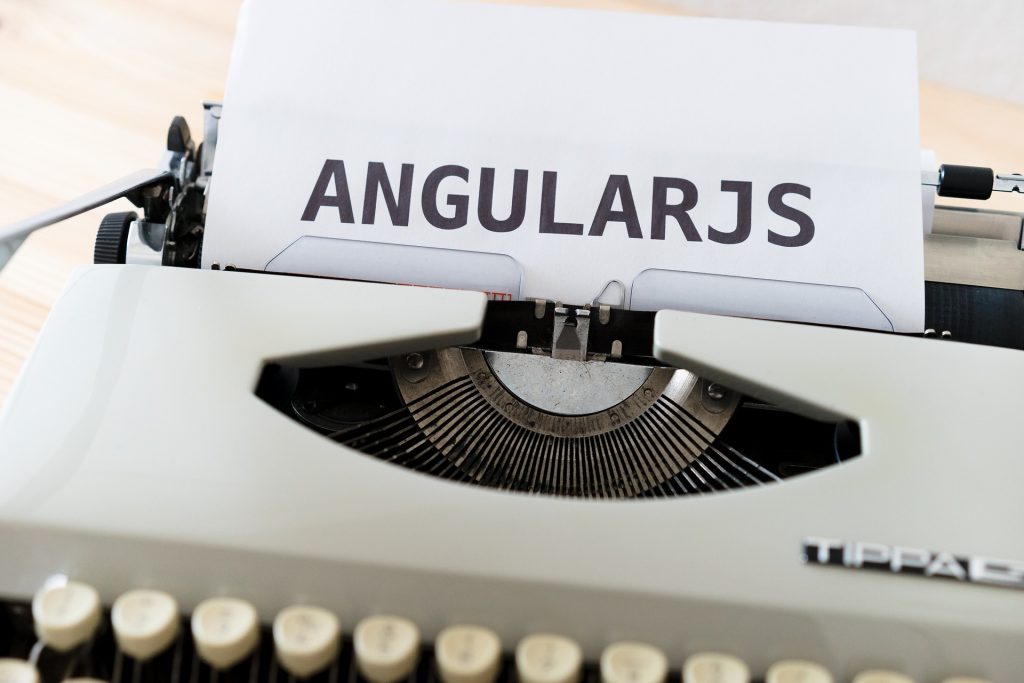
We then use the ng-repeat directive to iterate over an array of users and create a new row for each user. Within the ng-repeat directive, we define a user variable that represents each item in the users array.
Inside each row, we use AngularJS expressions (in double curly braces {{ }}) to display the name and email properties of each user.
To use this table, we must define the users array in our controller or service. Here’s an example:
angular.module('myApp', [])
.controller('myController', function($scope) {
$scope.users = [
{ name: 'John', email: 'john@example.com' },
{ name: 'Mary', email: 'mary@example.com' },
{ name: 'Peter', email: 'peter@example.com' }
];
});
In the above example, we define a new module called myApp and attach a controller to it called myController. We define an array of users in the controller, which contains some sample data.
We then use the $scope object to make the users array available to the view. This allows us to display the data in the table using the ng-repeat directive.
AngularJS Selection Boxes
In AngularJS, select boxes (also known as dropdown menus or elements) can be easily created and manipulated using the built-in ng-options directive. Here’s an example of how to create a select box using AngularJS:
<select ng-model="selectedColor" ng-options="color.name for color in colors"></select>
In the above example, we create a basic select box element and use the ng-model directive to bind the selected value to a variable called selectedColor.
We also use the ng-options directive to populate the select box with data from an array called colors. In this example, colors is an array of objects that have name and value properties:
angular.module('myApp', [])
.controller('myController', function($scope) {
$scope.colors = [
{ name: 'Red', value: '#FF0000' },
{ name: 'Green', value: '#00FF00' },
{ name: 'Blue', value: '#0000FF' }
];
});
In the above example, we define a new module called myApp and attach a controller to it called myController. We define an array of colors in the controller, which contains some sample data.
We then use the $scope object to make the colors array available to the view. This allows us to populate the select box using the ng-options directive.
SQL in AngularJS
AngularJS is a front-end web development framework and does not natively support SQL, which is a language used to manage relational databases. However, AngularJS can be used in conjunction with server-side technologies that do support SQL, such as PHP, Java, or Node.js, to build applications that interact with databases.
Here’s an example of how to use AngularJS with PHP and MySQL to retrieve data from a database:
To connect to the database and get data, first make a PHP script:
<?php
$servername = "localhost";
$username = "username";
$password = "password";
$dbname = "myDB";
// Create connection
$conn = new mysqli($servername, $username, $password, $dbname);
// Check connection
if ($conn->connect_error) {
die("Connection failed: " . $conn->connect_error);
}
// Retrieve data
$sql = "SELECT * FROM users";
$result = $conn->query($sql);
$data = array();
if ($result->num_rows > 0) {
while ($row = $result->fetch_assoc()) {
$data[] = $row;
}
}
// Send response
header('Content-Type: application/json');
echo json_encode($data);
$conn->close();
?>
In this example, we have created a PHP script that connects to a MySQL database and retrieves data from a table named users. The data is stored in an array and then encoded as JSON before being sent back to the client.
Next, create an AngularJS controller to retrieve the data from the PHP script and display it in the view:
<div ng-app="myApp" ng-controller="myController">
<ul>
<li ng-repeat="user in users">{{ user.name }}</li>
</ul>
</div>
var app = angular.module('myApp', []);
app.controller('myController', function($scope, $http) {
$http.get('/getUsers.php')
.then(function(response) {
$scope.users = response.data;
}, function(response) {
console.log('Error retrieving users: ' + response.statusText);
});
});
In this example, we have defined an AngularJS controller named “myController” which uses the $http service to make a GET request to the PHP script that retrieves the data from the database. Once the data is fetched, it is stored in the $scope.users property and displayed in the view using the ng-repeat directive.
HTML DOM in AngularJS
In AngularJS, the HTML DOM (Document Object Model) is used to manipulate the structure and content of web pages dynamically. AngularJS provides several built-in directives and services that allow developers to interact with the HTML DOM in a declarative and intuitive way.
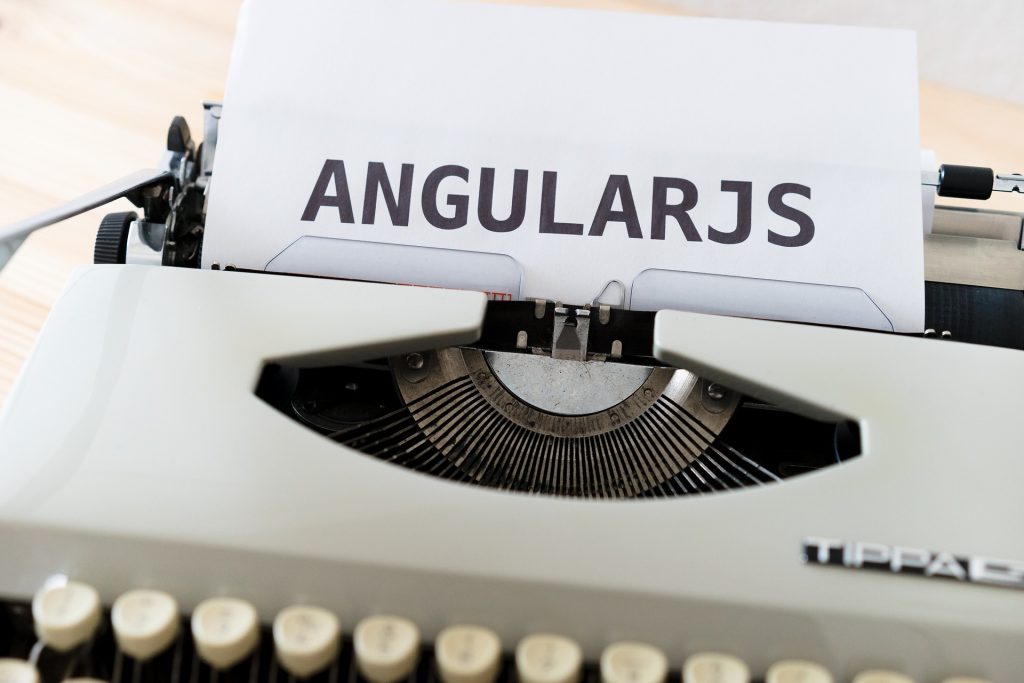
Here are some examples of how to use AngularJS directives and services to manipulate the HTML DOM:
1. ng-show and ng-hide:
The ng-show and ng-hide directives can be used to show or hide an element based on the value of a boolean expression. For example:
<div ng-show="showElement">This element is shown</div>
<div ng-hide="hideElement">This element is hidden</div>
In this example, the first element will be displayed if the showElement variable is true, and the second element will be hidden if the hideElement variable is true.\
2. ng-class:
The ng-class directive can be used to dynamically set the CSS classes of an element based on the value of an expression. For example:
<div ng-class="{ 'alert': isError, 'success': !isError }">This element has a dynamic class</div>
In this example, the alert class will be applied to the element if the isError variable is true, and the success class will be applied if the isError variable is false.
3. $timeout:
The $timeout service can be used to delay the execution of a function by a specified number of milliseconds. For example:
<button ng-click="showMessage()">Click me</button>
<div ng-show="message">{{ message }}</div>
app.controller('myController', function($scope, $timeout) {
$scope.showMessage = function() {
$scope.message = 'Hello, world!';
$timeout(function() {
$scope.message = '';
}, 5000);
};
});
In this example, clicking the button will show a message for 5 seconds using the ng-show directive and the $timeout service. The $timeout function is used to clear the message after 5 seconds.
Events in AngularJS
In AngularJS, events are used to handle user interactions and respond to changes in the application state. AngularJS provides several built-in directives and services for handling events.
Here are some examples of how to use AngularJS events:
1. ng-click:
To handle click events on an element, use the ng-click directive. For instance:
<button ng-click="buttonClicked()">Click me</button>
app.controller('myController', function($scope) {
$scope.buttonClicked = function() {
alert('Button clicked!');
};
});
In this example, clicking the button will call the buttonClicked() function and display an alert box.
2. ng-change:
The ng-change directive can be used to handle changes to the value of an input element. For example:
<input type="text" ng-model="name" ng-change="nameChanged()">
app.controller('myController', function($scope) {
$scope.nameChanged = function() {
console.log('Name changed: ' + $scope.name);
};
});
In this example, changing the value of the input element will call the nameChanged() function and log a message to the console.
3. $broadcast and $on:
The $broadcast and $on services can be used to send and receive events between different parts of the application. For example:
<button ng-click="sendMessage()">Send message</button>
app.controller('myController', function($scope, $rootScope) {
$scope.sendMessage = function() {
$rootScope.$broadcast('myEvent', { data: 'Hello, world!' });
};
});
app.controller('myOtherController', function($scope) {
$scope.$on('myEvent', function(event, args) {
console.log('Received message: ' + args.data);
});
});
In this example, clicking the button in the first controller will send a message using the $broadcast service. The second controller has registered a listener using the $on
service and will receive the message and log it to the console.