In Django, you can display data in your views and templates using various techniques and template tags. Here are some common approaches to display data in Django:
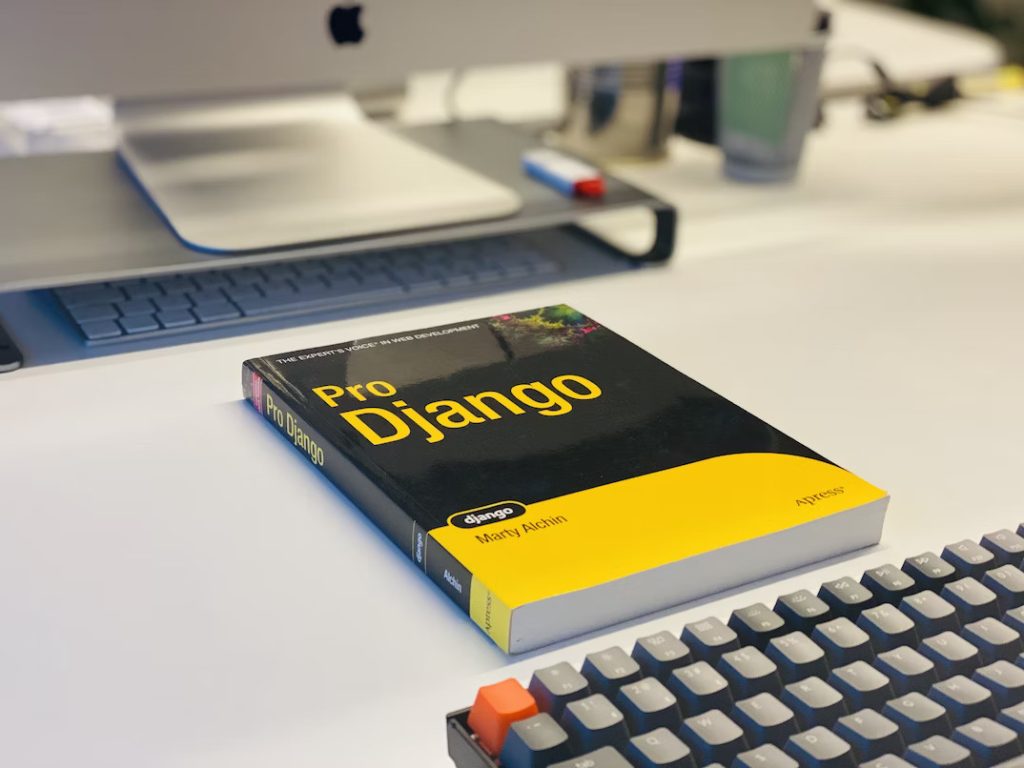
1. Passing Data to Templates:
In your Django view, you can pass data to a template by rendering the template with a Context or RequestContext object. You can include the data as variables in the context dictionary.
Example in views.py:
from django.shortcuts import render
def my_view(request):
data = {
'name': 'John Doe',
'age': 30,
}
return render(request, 'my_template.html', context=data)
In the above example, the data dictionary contains the variables name
and age
. The dictionary is passed as the context argument to the render function, and the template ‘my_template.html’ is rendered with that context.
2. Accessing Data in Templates:
Once the data is passed to the template, you can access it using template variables enclosed in double curly braces ({{}}).
Example in my_template.html:
<p>Name: {{ name }}</p>
<p>Age: {{ age }}</p>
In the template, name and age are accessed as template variables using {{}}. The corresponding values will be substituted in the rendered HTML.
3. Looping Over Data:
If you have a list or queryset of data, you can loop over it in the template using the {% for %} template tag.
Example in my_template.html:
<ul>
{% for item in items %}
<li>{{ item }}</li>
{% endfor %}
</ul>
In this example, the {% for %} tag is used to iterate over the items list or queryset. Each item is displayed as a list item (<li>) within an unordered list (<ul>).
4. Conditional Rendering:
You can use the {% if %} template tag to conditionally render content based on the value of a variable.
Example in my_template.html:
{% if age >= 18 %}
<p>Adult</p>
{% else %}
<p>Minor</p>
{% endif %}
In this example, the {% if %} tag checks if the age variable is greater than or equal to 18. Based on the condition, either the “Adult” or “Minor” paragraph will be rendered.
Template for Django Prepare
In Django, the term “template” refers to a file containing HTML markup and optional Django template tags. Templates are used to separate the presentation logic from the Python code in views, allowing you to dynamically generate HTML pages.
To prepare a template in Django, you typically follow these steps:
1. Create a Template File:
Make a template file with an.html extension first. Django templates are often kept in the application’s templates directory as a matter of tradition. If this directory is absent, you can create it.
Example template file (my_template.html):
<!DOCTYPE html>
<html>
<head>
<title>My Page</title>
</head>
<body>
<h1>Hello, {{ name }}!</h1>
</body>
</html>
In this example, the template file contains basic HTML markup with a placeholder ({{ name }}) that will be replaced with a dynamic value when the template is rendered.
2. Prepare the Template in the View:
In your Django view, you’ll need to load and prepare the template for rendering. This involves using the loader and render functions from Django’s template module.
Example in views.py:
from django.shortcuts import render
from django.template import loader
def my_view(request):
template = loader.get_template('my_template.html')
context = {'name': 'John Doe'}
rendered_template = template.render(context, request)
return HttpResponse(rendered_template)
Add Link to Details Django
In Django, adding a link to a details page typically involves creating a URL pattern, a view function, and a template to render the details page. Here’s a step-by-step guide with examples to help you understand the process.
1. Define the URL pattern:
Create a URL pattern in the urls.py file of your Django project that will capture the information required to recognize the details page. The object or entity you wish to display details about should be uniquely identified by a parameter in this pattern. As an demonstration, consider the following:
from django.urls import path
from . import views
urlpatterns = [
# Other URL patterns...
path('details/<int:pk>/', views.details_view, name='details'),
]
In this example, the URL pattern captures an integer parameter (<int:pk>) representing the primary key (pk) of the object.
2. Create a view function:
Create a view function in the views.py file of your Django application that renders the details template and obtains the object using the specified primary key. Here’s an demonstration:
from django.shortcuts import render, get_object_or_404
from .models import YourModel
def details_view(request, pk):
obj = get_object_or_404(YourModel, pk=pk)
return render(request, 'yourapp/details.html', {'object': obj})
In this example, the details_view function uses get_object_or_404 to retrieve the object from the database based on the provided primary key. It then passes the object to the details.html template.
3. Create a template for the details page:
Make a template file called details.html and place it in the templates directory of your project. The details page will be defined by this template’s structure and content. Here is a basic demonstration:
<h1>{{ object.title }}</h1>
<p>{{ object.description }}</p>
<!-- Other details you want to display -->
In this example, the template displays the title and description of the object, but you can include any additional details as needed.
4. Adding the link:
In your application’s relevant template file where you want to add the link, use the url template tag with the name of the URL pattern you defined earlier. Here’s an example:
<a href="{% url 'details' object.id %}">View Details</a>
In this example, the url template tag generates the URL based on the provided name (details) and the primary key (object.id). The link will lead to the details page for the corresponding object.
Make sure to replace ‘yourapp’ and ‘YourModel’ with the appropriate names for your Django app and model.
Add Master Template in Django
In Django, a master template (also known as a base template or layout template) is a template that serves as the foundation for other templates in your project. It contains common elements, such as the HTML structure, header, footer, and navigation menu, that are shared across multiple pages. Here’s a step-by-step guide with examples to help you understand how to create and use a master template in Django.
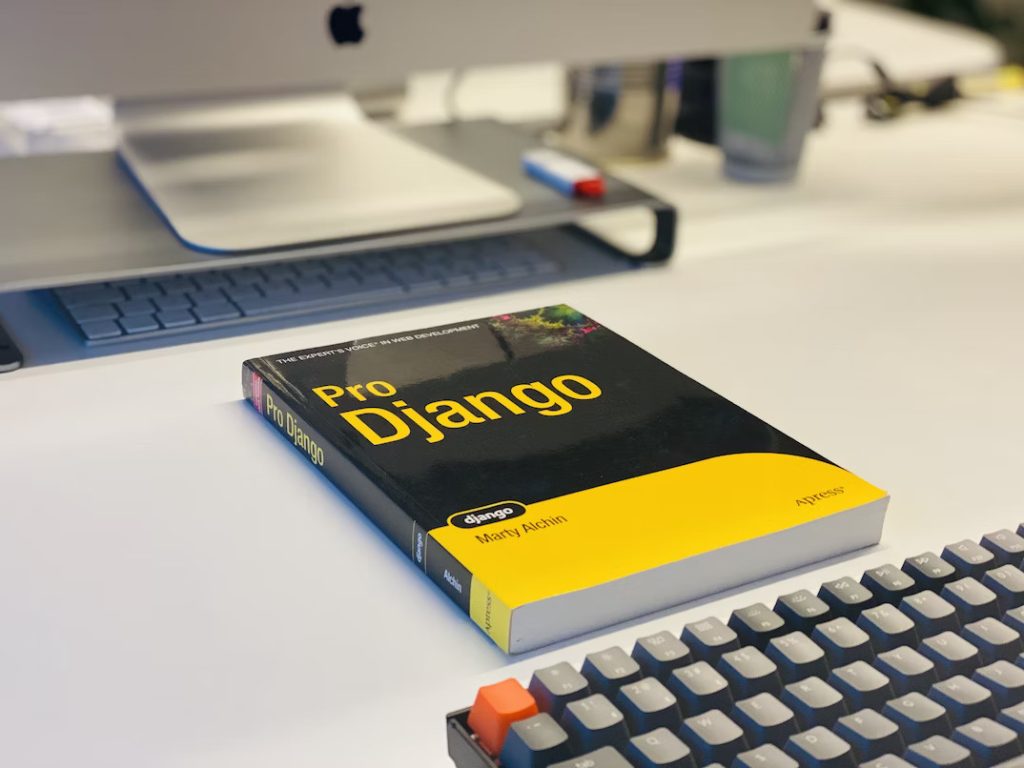
1. Create a base template:
In your Django project’s templates directory, create a file called base.html (or any other suitable name) to serve as your master template. This file will contain the common HTML structure and elements. Here’s an example:
<!-- base.html -->
<!DOCTYPE html>
<html>
<head>
<title>{% block title %}{% endblock %}</title>
<!-- Additional CSS and meta tags -->
</head>
<body>
<header>
<!-- Header content -->
</header>
<nav>
<!-- Navigation menu content -->
</nav>
<main>
{% block content %}
<!-- Content of child templates goes here -->
{% endblock %}
</main>
<footer>
<!-- Footer content -->
</footer>
</body>
</html>
In this instance, the placeholders (% block% tags) for the title, text, and other common components are provided by the base.html template.
2. Extend the base template:
In your child templates, you can extend the base.html template by inheriting its structure and overriding specific blocks with unique content. To extend the base template, use the {% extends %} template tag and define the blocks you want to override. Here’s an example:
<!-- child_template.html -->
{% extends 'base.html' %}
{% block title %}
Child Template Title
{% endblock %}
{% block content %}
<h1>Welcome to the Child Template!</h1>
<p>This is the content specific to the child template.</p>
{% endblock %}
By using {% extends%} the child_template.html template in this example extends the base.html template. For the child template, it overrides the {% block title%} and {% block content%} blocks with its own original content.
3. Rendering the child template:
To render the child template along with the base template, you can use Django’s {% extends %} and {% block %} tags. Here’s an example using Django views and the render() function:
from django.shortcuts import render
def my_view(request):
return render(request, 'child_template.html')
The child_template.html template, which builds on the base.html template in our example, is rendered by the my_view function. The structure specified in the base template will automatically be combined with the content of the child template by Django.
By using this approach, you can create a consistent layout across your Django project by reusing the base template and customizing specific sections as needed.
Add Main Index Page in Django
In Django, the main index page typically serves as the landing page or homepage of your web application. It’s the initial page users see when they visit your site. Here’s a step-by-step guide with examples to help you create and display a main index page in Django.
1. Create a template for the index page:
In your Django app’s templates directory, create a file called index.html (or any other suitable name) to define the structure and content of your main index page. Here’s a simple example:
<!-- index.html -->
<!DOCTYPE html>
<html>
<head>
<title>My Website - Home</title>
</head>
<body>
<h1>Welcome to My Website!</h1>
<p>This is the main index page of my web application.</p>
</body>
</html>
In this instance, a header and a paragraph with a welcome message are included in the index.html template’s basic HTML syntax.
2. Define a view function for the index page:
Define a view function in your Django app’s views.py file that renders the index.html template. Here’s an demonstration:
from django.shortcuts import render
def index_view(request):
return render(request, 'index.html')
In this example, the index_view function uses the render() function to render the index.html template.
3. Configure the URL pattern:
Configure a URL pattern that corresponds to the index_view function in your Django project’s urls.py file. Here’s an demmonstration:
from django.urls import path
from . import views
urlpatterns = [
path('', views.index_view, name='index'),
# Other URL patterns...
]
In this example, the empty string ''
as the URL pattern matches the root URL of your application. It associates the URL with the index_view function.
4. Display the index page:
Finally, you can access the main index page by visiting the root URL of your Django application. For example, if you are running your Django project locally, you can navigate to http://localhost:8000/ in your web browser to see the index page.
404 (page not found) in Django
In Django, a 404 page is displayed when a requested URL is not found or does not exist. It provides a user-friendly message indicating that the requested resource could not be located. Django includes a default 404 page, but you can also customize it to match the design and styling of your application. Here’s how you can work with Django’s 404 page:
1. Customizing the 404 page:
Create a template file called 404.html in your app’s templates directory to modify the 404 page. When a 404 error occurs, this template is presented. Here’s an illustration:
<!-- 404.html -->
<!DOCTYPE html>
<html>
<head>
<title>Page Not Found</title>
</head>
<body>
<h1>Oops! Page Not Found</h1>
<p>The requested page could not be found.</p>
</body>
</html>
The 404.html template in this example displays a basic message stating that the page could not be found.
2. Testing the 404 page:
To test the 404 page, you can intentionally visit a URL that does not exist in your Django application. Django’s development server will automatically display the custom 404 page you created.
3. Raising a 404 error in views:
In your view functions, you can manually raise a 404 error when certain conditions are not met. This can be useful when you want to handle specific cases where a requested resource is not available. Here’s an example:
from django.http import Http404
def my_view(request):
if some_condition:
raise Http404("This page does not exist")
else:
# Process the request as normal
pass
In this example, when the some_condition is not met, the view raises a Http404 exception with a custom error message. Django will handle the exception and render the 404 page.
4. Using get_object_or_404:
Django provides a shortcut function called get_object_or_404 that retrieves an object from the database based on specified conditions. If the object is not found, it automatically raises a 404 error. Here’s an example:
from django.shortcuts import get_object_or_404
from .models import MyModel
def my_view(request, pk):
obj = get_object_or_404(MyModel, pk=pk)
# Process the object if found
pass
In this example, the get_object_or_404 function tries to retrieve an object of MyModel with the specified primary key (pk). If the object does not exist, it raises a 404 error.
By customizing the 404 page and utilizing the appropriate techniques, you can handle cases where URLs are not found or resources are unavailable in your Django application, providing a user-friendly experience for your users.
Add a Test View to Django
To add a test view in Django, you need to define a view function, create a URL pattern, and optionally create a template to render the view’s content. Here’s a step-by-step guide with examples:
1. Define the view function:
Define a view function in your Django app’s views.py file that will handle the request and provide the response. demonstration:
from django.http import HttpResponse
def test_view(request):
return HttpResponse("This is a test view.")
In this example, the test_view function takes a request parameter and returns an HttpResponse object with the desired content. You can customize the content based on your requirements.
2. Create a URL pattern:
In your Django app’s urls.py file, create a URL pattern that maps the desired URL path to the test_view function. Here’s an example:
from django.urls import path
from . import views
urlpatterns = [
# Other URL patterns...
path('test/', views.test_view, name='test'),
]
In this example, the URL pattern ‘test/’ maps to the test_view function. The name=’test’ argument assigns a name to the URL pattern, allowing you to refer to it by name in other parts of your code.
3. (Optional) Create a template:
If you want to render a template for the test view, create a template file in your app’s templates directory. For example, create a file called test.html:
<!-- test.html -->
<!DOCTYPE html>
<html>
<head>
<title>Test View</title>
</head>
<body>
<h1>This is a test view.</h1>
<!-- Other content as needed -->
</body>
</html>
4. Rendering the view:
To render the view, you need to either include the URL in a template or access it directly via the defined URL path. Here are two examples:
a. Including the URL in a template:
In a template file, you can use the {% url %} template tag to generate the URL for the test view. Here’s an example:
<!-- my_template.html -->
<a href="{% url 'test' %}">Go to Test View</a>
In this example, the {% url ‘test’ %} tag generates the URL based on the provided name (‘test’), and the link will lead to the test view when clicked.
b. Accessing the URL directly:
If you know the URL path for the test view, you can directly access it in your web browser. For example, if you are running your Django project locally, you can navigate to http://localhost:8000/test/ to see the test view.