In Django, URLs (Uniform Resource Locators) are used to map specific URLs or URL patterns to corresponding views in your Django project. URLs define the structure of your web application and determine how the user’s requests are routed to the appropriate views for processing.
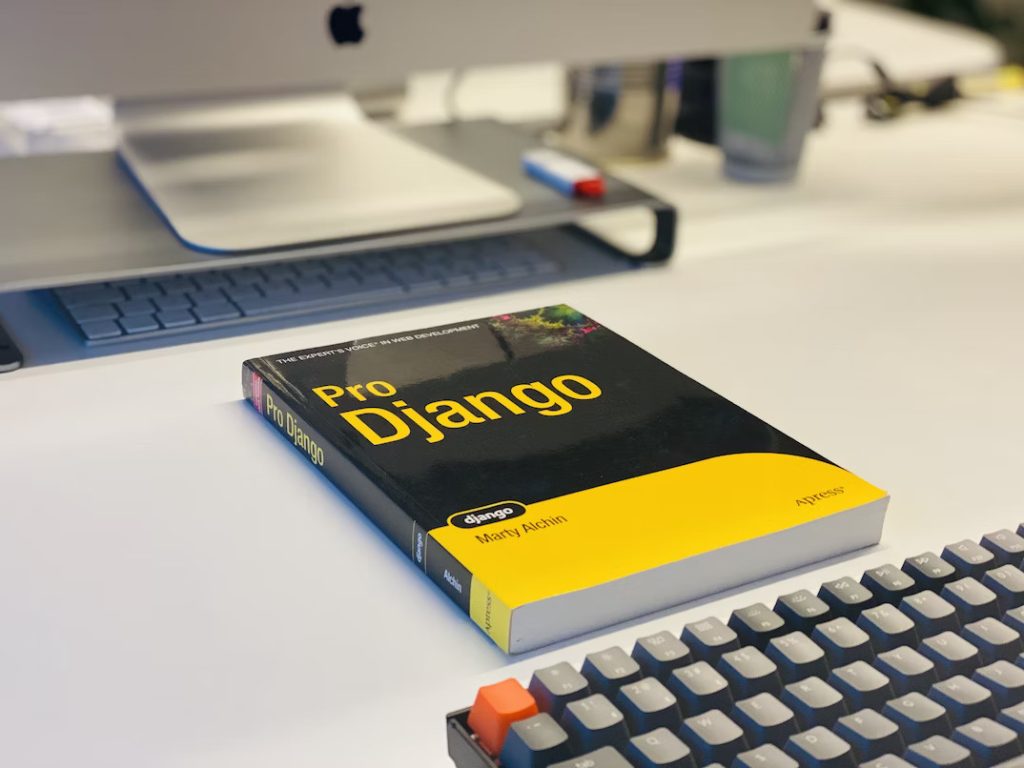
URL configuration in Django involves creating a set of URL patterns in a Python module, typically named urls.py. This module is usually present in the project’s root directory and in each Django app’s directory. The URL patterns are defined using regular expressions or string patterns and are associated with specific views.
Here’s an overview of how URLs work in Django:
1. Project-level URLs:
You provide the top-level URL patterns that apply to the whole project in the root urls.py file. The URLs of the many apps included in the project are frequently included in these patterns. For instance:
from django.urls import include, path
urlpatterns = [
path('admin/', admin.site.urls),
path('app/', include('myapp.urls')),
]
In this example, the URLs starting with “admin/” are routed to Django’s built-in admin views, while the URLs starting with “app/” are passed to the urls.py file of the myapp app.
2. App-level URLs:
The urls.py file, which is unique to each Django app, is where you define the URL patterns exclusive to that app. Using the include() method, these URL patterns are added to the project-level urls.py file. Here’s an demonstration:
from django.urls import path
from . import views
urlpatterns = [
path('hello/', views.hello_view, name='hello'),
# other app-specific URLs
]
In this example, the URL pattern “hello/” is associated with the hello_view function in the app’s views.py module. The name parameter provides a unique name for the URL pattern, which can be used for reversing URLs.
3. URL Parameters:
Django allows you to capture dynamic segments of a URL and pass them as parameters to the associated view. You can use angle brackets (< >) to define URL parameters in your URL patterns. Here’s an example:
from django.urls import path
from . import views
urlpatterns = [
path('books/<int:book_id>/', views.book_detail, name='book_detail'),
# other app-specific URLs
]
In this example, the URL pattern “books/int:book_id/” captures an integer value as book_id and passes it as a parameter to the book_detail view.
4. Namespaced URLs:
Django allows you to namespace your URLs to avoid naming conflicts. This is particularly useful when multiple apps have similar URL patterns. To create a namespace, define the app_name attribute in the app’s urls.py file and provide a unique name. Here’s an example:
app_name = 'myapp'
urlpatterns = [
# app-specific URLs
]
In the project-level urls.py, you can then include the app URLs with the specified namespace:
path('app/', include(('myapp.urls', 'myapp'), namespace='myapp')),
This allows you to refer to specific URLs using the app_name:URL_name syntax, which helps differentiate between similar URLs from different apps.
Templates for Django
In Django, templates are used to generate dynamic HTML pages by combining static HTML markup with dynamic data from views. Templates allow you to separate the presentation logic from the application logic, making it easier to maintain and update your web application.
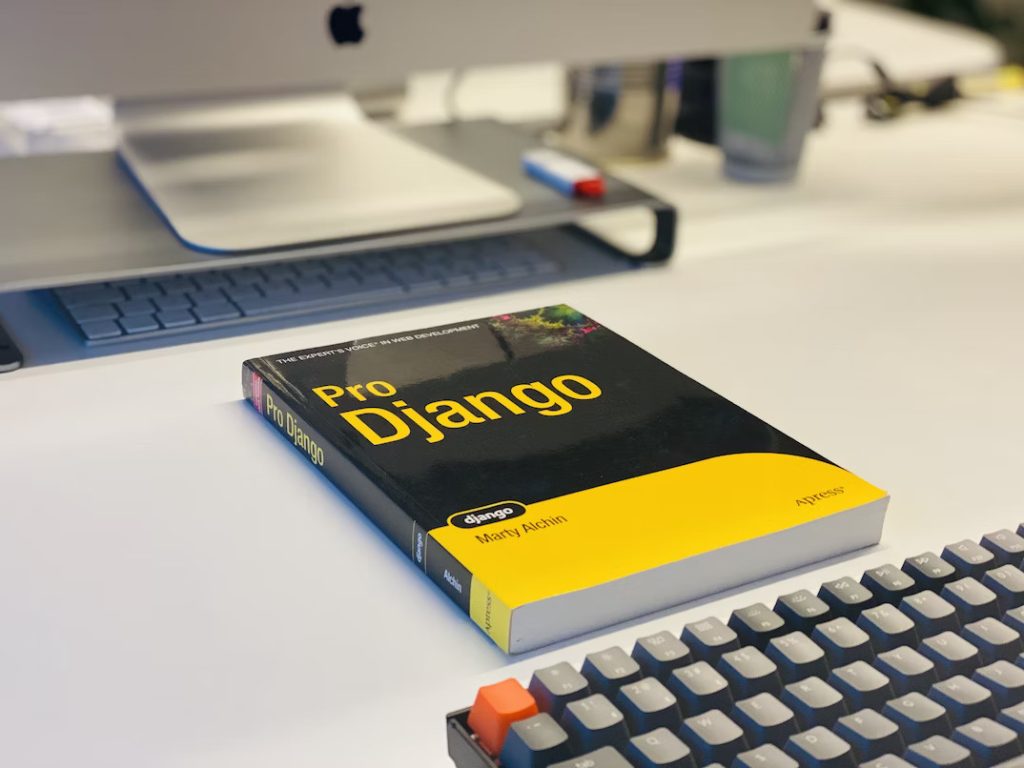
Django’s template system uses its own template language, which is a simplified syntax that allows you to insert variables, perform loops and conditionals, and include reusable template components. Here’s an overview of Django templates:
1. Template Files:
Templates are kept in their own files with the html extension. These files are generally stored in a templates directory inside each Django project. If your app is called myapp, for instance, you may have a template file in myapp/templates/myapp/template_name.html.
2. Template Language:
Django’s template language provides a set of tags, filters, and variables that enable you to manipulate and display data within your templates. Some commonly used constructs include:
- Variables: These are represented by double curly braces ({{ variable_name }}) and allow you to output dynamic values in your template.
- Tags: Tags are enclosed in {% %} and provide control structures like loops, conditionals, and template inheritance.
- Filters: Filters change output or variables. They may format, filter, and sort data, and they are applied by using the pipe symbol (|).
Here’s an example of a template that uses variables, tags, and filters:
<h1>Hello, {{ name|title }}!</h1>
{% if age >= 18 %}
<p>You are an adult.</p>
{% else %}
<p>You are a minor.</p>
{% endif %}
<ul>
{% for item in items %}
<li>{{ item }}</li>
{% endfor %}
</ul>
In this example, the template receives name, age, and items as context variables and uses tags and filters to conditionally render different parts of the HTML.
3. Rendering Templates:
To render a template, you typically pass it a context, which is a dictionary containing the variables and values to be used in the template. Django’s views are responsible for rendering templates and passing the necessary context.
Here’s an example of rendering a template in a view:
from django.shortcuts import render
def my_view(request):
name = "John"
age = 25
items = ["Apple", "Banana", "Orange"]
context = {
'name': name,
'age': age,
'items': items
}
return render(request, 'myapp/template_name.html', context)
In this example, the render function takes the request object, the path to the template file, and the context dictionary. It processes the template and returns an HTTP response with the rendered HTML.
4. Template Inheritance:
Django templates support inheritance, allowing you to create a base template with common elements and extend it in other templates. This helps you maintain consistency across multiple pages. The base template defines blocks that child templates can override with their own content. Here’s an example:
<!-- base.html -->
<html>
<head>
<title>{% block title %}My Site{% endblock %}</title>
</head>
<body>
{% block content %}
{% endblock %}
</body>
</html>
<!-- child.html -->
{% extends "base.html" %}
{% block title %}My Page{%
Models for Django
In Django, models are Python classes that represent database tables or collections. They define the structure and behavior of the data in the database and provide an interface to interact with the data using object-oriented programming concepts.
Here’s a definition and an example of Django models:
Definition:
A database table or collection is represented by a Django model, which is a subclass of django.db.models. Model. Along with any relevant information or actions, it defines fields that describe the table’s columns or characteristics.
Example:
Let’s say we want to create a simple model to represent a blog post. Here’s how you would define a Django model for it:
from django.db import models
class BlogPost(models.Model):
title = models.CharField(max_length=200)
content = models.TextField()
published_date = models.DateTimeField(auto_now_add=True)
In this example:
- From django.db, we import the models module.
- We create the class BlogPost, which is an inheritor of models.Model.
- Inside the class, we define three fields: title, content, and published_date.
- The title field is a CharField with a 200 character limit.
- The content field is a TextField for larger text content.
- The published_date field is a DateTimeField that automatically sets the current date and time when the object is created (auto_now_add=True).
This model defines the structure of a blog post, including its title, content, and published date. Each field in the model corresponds to a column in the database table that will store the blog post data.
To use this model, you need to perform database migrations. Migrations are the process of synchronizing your models with the database schema. You can create migrations using the makemigrations command and apply them using the migrate command in Django’s manage.py file.
Once the migrations are applied, you can use the model to create, retrieve, update, and delete instances of blog posts in your views or other parts of your Django application.
Django models offer many additional features and field types to handle relationships, data validation, querying, and more. You can refer to Django’s documentation for a comprehensive guide on models and their functionalities.
Insert Data in Django
In Django, you can insert data into a database using models and Django’s Object-Relational Mapping (ORM) system. The ORM provides an abstraction layer that allows you to interact with the database using Python code and Django models.
To insert data into a database using Django, you typically follow these steps:
1. The model should be created as an instance.
2. Set the attributes or fields of the model instance with the desired values.
3. Database saving for the model instance.
Here’s an example that demonstrates how to insert data into a database using Django models:
Assuming we have a model called Book that represents a book with a title and an author, defined as follows:
from django.db import models
class Book(models.Model):
title = models.CharField(max_length=200)
author = models.CharField(max_length=100)
To insert a book into the database, you can perform the following steps:
1. Create an instance of the Book model after importing it:
from myapp.models import Book
book = Book()
2. Set the attributes or fields of the book instance:
book.title = "The Great Gatsby"
book.author = "F. Scott Fitzgerald"
3. Save the book instance to the database using the save() method:
book.save()
After calling save(), Django will generate the necessary SQL statements and execute them to insert the data into the corresponding database table.
You can also perform bulk insertions by creating multiple model instances and saving them in a loop. Here’s an example:You can also perform bulk insertions by creating multiple model instances and saving them in a loop. Here’s an example:
from myapp.models import Book
books_data = [
{'title': 'Book 1', 'author': 'Author 1'},
{'title': 'Book 2', 'author': 'Author 2'},
{'title': 'Book 3', 'author': 'Author 3'},
]
for data in books_data:
book = Book()
book.title = data['title']
book.author = data['author']
book.save()
In this example, we have a list of dictionaries representing book data. We iterate over each dictionary, create a new Book instance, set the attributes, and save the instance to the database.
By following these steps, you can insert data into a database using Django models and the ORM. Remember to import the necessary models, create instances, set the attributes, and call the save() method to persist the data in the database.
Update Data for Django
In Django, you can update data in a database using models and the Object-Relational Mapping (ORM) provided by Django. The ORM allows you to interact with the database using Python code and Django models.
To update data in a database using Django, you typically follow these steps:
1. Retrieve the existing record(s) from the database.
2. Modify the desired attribute(s) or field(s) of the model instance.
3. Save the database with the changed model instance.
Here’s an example that demonstrates how to update data in a database using Django models:
Assuming we have a model called Book
that represents a book with a title and an author, defined as follows:
from django.db import models
class Book(models.Model):
title = models.CharField(max_length=200)
author = models.CharField(max_length=100)
To update a book’s author in the database, you can perform the following steps:
1. Retrieve the book record(s) to be updated from the database. You can use Django’s query methods, such as get(), filter(), or all(), to retrieve the record(s) based on specific conditions. For example, to update a book with a particular title:
from myapp.models import Book
book = Book.objects.get(title="The Great Gatsby")
2. Modify the desired attribute(s) or field(s) of the book instance:
book.author = "F. Scott Fitzgerald"
After calling save(), Django will generate the necessary SQL statements and execute them to update the corresponding record(s) in the database.
You can also update multiple records in a similar way by retrieving a queryset of records based on specific conditions and iterating over them to update the desired attributes. Here’s an example:
from myapp.models import Book
books = Book.objects.filter(author="Author 1")
for book in books:
book.author = "New Author"
book.save()
In this example, we retrieve all books with the author “Author 1” using the filter() method. Then, we iterate over each book, update the author attribute, and save the updated book instance to the database.
By following these steps, you can update data in a database using Django models and the ORM. Retrieve the existing record(s), modify the desired attributes, and call the save() method to update the data in the database.
Delete Data in Django
In Django, you can delete data from a database using models and the Object-Relational Mapping (ORM) provided by Django. The ORM allows you to interact with the database using Python code and Django models.
To delete data from a database using Django, you typically follow these steps:
1. Retrieve the record(s) from the database that you want to delete.
2. Call the delete() method on the model instance(s) or queryset to delete the record(s) from the database.
Here’s an example that demonstrates how to delete data from a database using Django models:
Assuming we have a model called Book that represents a book with a title and an author, defined as follows:
from django.db import models
class Book(models.Model):
title = models.CharField(max_length=200)
author = models.CharField(max_length=100)
from django.db import models
class Book(models.Model):
title = models.CharField(max_length=200)
author = models.CharField(max_length=100)
To delete a book from the database, you can perform the following steps:
1. Retrieve the book record(s) to be deleted from the database. You can use Django’s query methods, such as get(), filter(), or all(), to retrieve the record(s) based on specific conditions. For example, to delete a book with a particular title:
from myapp.models import Book
book = Book.objects.get(title="The Great Gatsby")
2. Call the delete()
method on the book
instance to delete it from the database:
book.delete()
After calling delete(), Django will generate the necessary SQL statements and execute them to delete the corresponding record(s) from the database.
You can also delete multiple records by retrieving a queryset of records based on specific conditions and calling the delete() method on the queryset. Here’s an example:
from myapp.models import Book
books = Book.objects.filter(author="Author 1")
books.delete()
In this example, we retrieve all books with the author “Author 1” using the filter() method. Then, we call the delete() method on the books queryset to delete all matching records from the database.
By following these steps, you can delete data from a database using Django models and the ORM. Retrieve the record(s) to be deleted, and call the delete() method on the model instance(s) or queryset to remove the data from the database.
Update Model for Django
In Django, updating a model involves modifying the structure or behavior of an existing database table represented by the model. You can update a model by making changes to its fields, adding new fields, removing fields, or altering any other aspect of the model’s definition.
Here’s an overview of how to update a model in Django:
1. Make the necessary changes to the model’s definition.
2. Create a migration to record the changes.
3. To update the database schema, implement the migration.
Let’s walk through each step using an demonstration:
Assuming we have a model called Book that represents a book with a title and an author, defined as follows:
from django.db import models
class Book(models.Model):
title = models.CharField(max_length=200)
author = models.CharField(max_length=100)
To update the Book model, let’s say we want to add a new field called publication_date:
from django.db import models
class Book(models.Model):
title = models.CharField(max_length=200)
author = models.CharField(max_length=100)
publication_date = models.DateField()
After updating the model’s definition, we need to create a migration to record the changes. Django provides a command-line tool, manage.py, to create and apply migrations.
To create a migration for the model update, run the following command:
python manage.py makemigrations
This command analyzes the changes in the models and generates a migration file, which includes the instructions to update the database schema.
Once the migration file is created, you can apply it to the database using the following command:
python manage.py migrate
This command executes the necessary SQL statements to update the database schema according to the migration file. The migrate command ensures that the database schema and the model definitions are in sync.