To set up a React project, you’ll need to follow these steps:
1. Install Node.js: React requires Node.js, so if you don’t already have it installed, download and install it from the Node.js website.
2. Make a new project directory: In your terminal, create a new directory for your project and go to it.
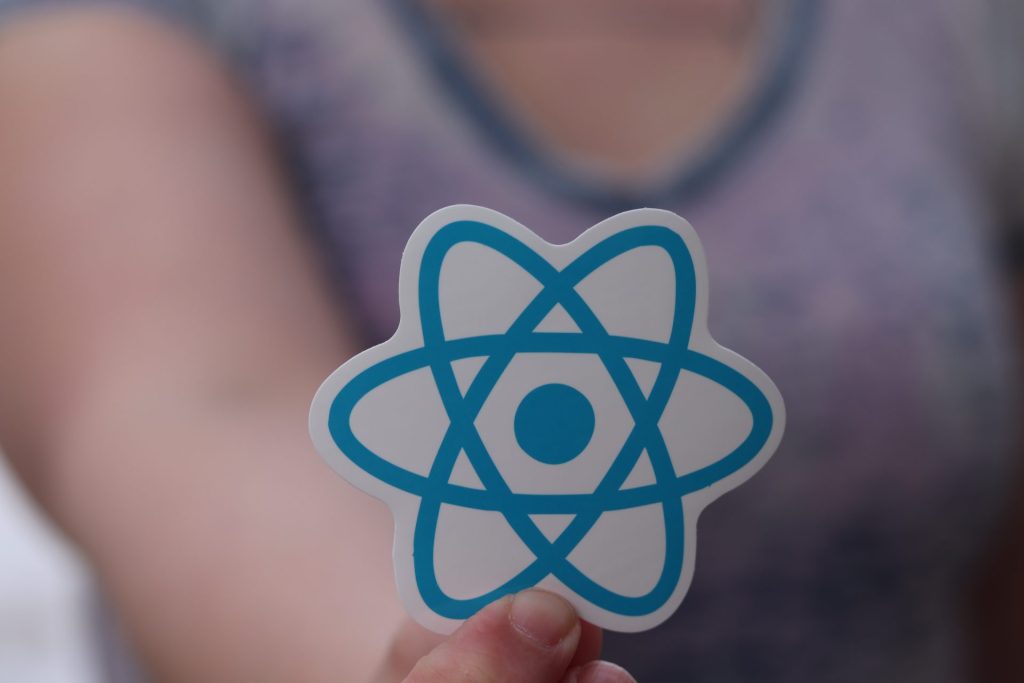
3. Set up the package.json file and start a new Node.js project: Run the following command to start a new Node.js project.
npm init -y
4. Install React and React DOM: Run the following command to install React and React DOM as dependencies:
npm install react react-dom
5. Set up a build process: You’ll need to set up a build process to compile your React code into JavaScript that can be run in a browser. There are several tools you can use for this, but one popular option is webpack. You’ll need to install webpack and some related plugins as dev dependencies:
npm install webpack webpack-cli webpack-dev-server babel-loader @babel/core @babel/preset-env @babel/preset-react html-webpack-plugin clean-webpack-plugin --save-dev
6. Create a basic React component: Create a new file in your project directory called index.js and add the following code to create a basic React component:
import React from 'react';
import ReactDOM from 'react-dom';
function App() {
return (
<div>
<h1>Hello, world!</h1>
</div>
);
}
ReactDOM.render(<App />, document.getElementById('root'));
7. Create an HTML file: Create a new file in your project directory called index.html and add the following code to create a basic HTML page:
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>My React App</title>
</head>
<body>
<div id="root"></div>
<script src="dist/main.js"></script>
</body>
</html>
8. Create a webpack configuration file: Create a new file in your project directory called webpack.config.js and add the following code:
const path = require('path');
const HtmlWebpackPlugin = require('html-webpack-plugin');
const { CleanWebpackPlugin } = require('clean-webpack-plugin');
module.exports = {
entry: './index.js',
output: {
filename: 'main.js',
path: path.resolve(__dirname, 'dist'),
},
module: {
rules: [
{
test: /\.js$/,
exclude: /node_modules/,
use: {
loader: 'babel-loader',
options: {
presets: ['@babel/preset-env', '@babel/preset-react'],
},
},
},
],
},
plugins: [
new CleanWebpackPlugin(),
new HtmlWebpackPlugin({
template: 'index.html',
}),
],
devServer: {
contentBase: './dist',
port: 3000,
},
};
9. Build and run the app: Run the following command to build the app and start the development server:
npx webpack serve --mode development
This will start a development server at http://localhost:3000, where you can view your app running.
You’ve now set up a basic React project. You can modify the App component in index.js to create your own React app.